mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2024-07-04 11:03:22 +00:00
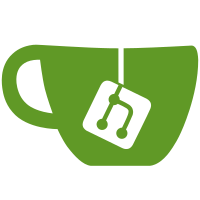
We currently generate the crafting item once when the upgrade is first created, and cache it for the duration of the game. As the item never changes throughout the game, and constructing a stack is a little expensive (we need to fire an event, etc...), the caching is worth having. However, some mods may register capabilities after we've constructed our ItemStack. This means the capability will be present on other items but not ours, meaning they are not considered equivalent, and thus the item cannot be equipped. In order to avoid this, we use compare items using their share-tag, like Forge's IngredientNBT. This means the items must still be "mostly" the same (same enchantements, etc...), but allow differing capabilities. See NillerMedDild/Enigmatica2Expert#655 for the original bug report - in this case, Astral Sourcery was registering the capability in init, but we construct upgrades just before then.
70 lines
2.2 KiB
Java
70 lines
2.2 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2019. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
|
|
package dan200.computercraft.shared;
|
|
|
|
import com.google.common.base.Preconditions;
|
|
import dan200.computercraft.ComputerCraft;
|
|
import dan200.computercraft.api.pocket.IPocketUpgrade;
|
|
import dan200.computercraft.shared.util.InventoryUtil;
|
|
import net.minecraft.item.ItemStack;
|
|
|
|
import javax.annotation.Nonnull;
|
|
import java.util.ArrayList;
|
|
import java.util.HashMap;
|
|
import java.util.List;
|
|
import java.util.Map;
|
|
|
|
public final class PocketUpgrades
|
|
{
|
|
private static final Map<String, IPocketUpgrade> upgrades = new HashMap<>();
|
|
|
|
public static void register( @Nonnull IPocketUpgrade upgrade )
|
|
{
|
|
Preconditions.checkNotNull( upgrade, "upgrade cannot be null" );
|
|
|
|
String id = upgrade.getUpgradeID().toString();
|
|
IPocketUpgrade existing = upgrades.get( id );
|
|
if( existing != null )
|
|
{
|
|
throw new IllegalStateException( "Error registering '" + upgrade.getUnlocalisedAdjective() + " pocket computer'. UpgradeID '" + id + "' is already registered by '" + existing.getUnlocalisedAdjective() + " pocket computer'" );
|
|
}
|
|
|
|
upgrades.put( id, upgrade );
|
|
}
|
|
|
|
|
|
public static IPocketUpgrade get( String id )
|
|
{
|
|
return upgrades.get( id );
|
|
}
|
|
|
|
public static IPocketUpgrade get( @Nonnull ItemStack stack )
|
|
{
|
|
if( stack.isEmpty() ) return null;
|
|
|
|
for( IPocketUpgrade upgrade : upgrades.values() )
|
|
{
|
|
ItemStack craftingStack = upgrade.getCraftingItem();
|
|
if( !craftingStack.isEmpty() && InventoryUtil.areItemsSimilar( stack, craftingStack ) )
|
|
{
|
|
return upgrade;
|
|
}
|
|
}
|
|
|
|
return null;
|
|
}
|
|
|
|
public static Iterable<IPocketUpgrade> getVanillaUpgrades()
|
|
{
|
|
List<IPocketUpgrade> vanilla = new ArrayList<>();
|
|
vanilla.add( ComputerCraft.PocketUpgrades.wirelessModem );
|
|
vanilla.add( ComputerCraft.PocketUpgrades.advancedModem );
|
|
vanilla.add( ComputerCraft.PocketUpgrades.speaker );
|
|
return vanilla;
|
|
}
|
|
}
|