mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2024-06-28 08:03:21 +00:00
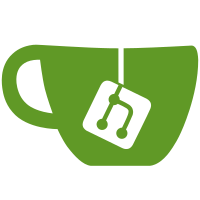
When creating a peripheral or custom Lua object, one must implement two methods: - getMethodNames(): String[] - Returns the name of the methods - callMethod(int, ...): Object[] - Invokes the method using an index in the above array. This has a couple of problems: - It's somewhat unwieldy to use - you need to keep track of array indices, which leads to ugly code. - Functions which yield (for instance, those which run on the main thread) are blocking. This means we need to spawn new threads for each CC-side yield. We replace this system with a few changes: - @LuaFunction annotation: One may annotate a public instance method with this annotation. This then exposes a peripheral/lua object method. Furthermore, this method can accept and return a variety of types, which often makes functions cleaner (e.g. can return an int rather than an Object[], and specify and int argument rather than Object[]). - MethodResult: Instead of returning an Object[] and having blocking yields, functions return a MethodResult. This either contains an immediate return, or an instruction to yield with some continuation to resume with. MethodResult is then interpreted by the Lua runtime (i.e. Cobalt), rather than our weird bodgey hacks before. This means we no longer spawn new threads when yielding within CC. - Methods accept IArguments instead of a raw Object array. This has a few benefits: - Consistent argument handling - people no longer need to use ArgumentHelper (as it doesn't exist!), or even be aware of its existence - you're rather forced into using it. - More efficient code in some cases. We provide a Cobalt-specific implementation of IArguments, which avoids the boxing/unboxing when handling numbers and binary strings.
67 lines
2.7 KiB
Java
67 lines
2.7 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2020. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
package dan200.computercraft.core.lua;
|
|
|
|
import dan200.computercraft.api.lua.IDynamicLuaObject;
|
|
import dan200.computercraft.api.lua.ILuaAPI;
|
|
|
|
import javax.annotation.Nonnull;
|
|
import javax.annotation.Nullable;
|
|
import java.io.InputStream;
|
|
|
|
/**
|
|
* Represents a machine which will execute Lua code. Technically this API is flexible enough to support many languages,
|
|
* but you'd need a way to provide alternative ROMs, BIOSes, etc...
|
|
*
|
|
* There should only be one concrete implementation at any one time, which is currently {@link CobaltLuaMachine}. If
|
|
* external mod authors are interested in registering their own machines, we can look into how we can provide some
|
|
* mechanism for registering these.
|
|
*
|
|
* This should provide implementations of {@link dan200.computercraft.api.lua.ILuaContext}, and the ability to convert
|
|
* {@link IDynamicLuaObject}s into something the VM understands, as well as handling method calls.
|
|
*/
|
|
public interface ILuaMachine
|
|
{
|
|
/**
|
|
* Inject an API into the global environment of this machine. This should construct an object, as it would for any
|
|
* {@link IDynamicLuaObject} and set it to all names in {@link ILuaAPI#getNames()}.
|
|
*
|
|
* Called before {@link #loadBios(InputStream)}.
|
|
*
|
|
* @param api The API to register.
|
|
*/
|
|
void addAPI( @Nonnull ILuaAPI api );
|
|
|
|
/**
|
|
* Create a function from the provided program, and set it up to run when {@link #handleEvent(String, Object[])} is
|
|
* called.
|
|
*
|
|
* This should destroy the machine if it failed to load the bios.
|
|
*
|
|
* @param bios The stream containing the boot program.
|
|
* @return The result of loading this machine. Will either be OK, or the error message when loading the bios.
|
|
*/
|
|
MachineResult loadBios( @Nonnull InputStream bios );
|
|
|
|
/**
|
|
* Resume the machine, either starting or resuming the coroutine.
|
|
*
|
|
* This should destroy the machine if it failed to execute successfully.
|
|
*
|
|
* @param eventName The name of the event. This is {@code null} when first starting the machine. Note, this may
|
|
* do nothing if it does not match the event filter.
|
|
* @param arguments The arguments for this event.
|
|
* @return The result of loading this machine. Will either be OK, or the error message that occurred when
|
|
* executing.
|
|
*/
|
|
MachineResult handleEvent( @Nullable String eventName, @Nullable Object[] arguments );
|
|
|
|
/**
|
|
* Close the Lua machine, aborting any running functions and deleting the internal state.
|
|
*/
|
|
void close();
|
|
}
|