mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2025-07-03 10:32:52 +00:00
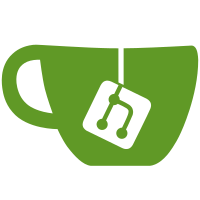
illuaminate does not handle Java files, for obvious reasons. In order to get around that, we have a series of stub files within /doc/stub which mirrored the Java ones. While this works, it has a few problems: - The link to source code does not work - it just links to the stub file. - There's no guarantee that documentation remains consistent with the Java code. This change found several methods which were incorrectly documented beforehand. We now replace this with a custom Java doclet[1], which extracts doc comments from @LuaFunction annotated methods and generates stub-files from them. These also contain a @source annotation, which allows us to correctly link them back to the original Java code. There's some issues with this which have yet to be fixed. However, I don't think any of them are major blockers right now: - The custom doclet relies on Java 9 - I think it's /technically/ possible to do this on Java 8, but the API is significantly uglier. This means that we need to run javadoc on a separate JVM. This is possible, and it works locally and on CI, but is definitely not a nice approach. - illuaminate now requires the doc stubs to be generated in order for the linter to pass, which does make running the linter locally much harder (especially given the above bullet point). We could notionally include the generated stubs (or at least a cut down version of them) in the repo, but I'm not 100% sure about that. [1]: https://docs.oracle.com/javase/9/docs/api/jdk/javadoc/doclet/package-summary.html
132 lines
3.5 KiB
Java
132 lines
3.5 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2020. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
package dan200.computercraft.shared.peripheral.printer;
|
|
|
|
import dan200.computercraft.api.lua.IArguments;
|
|
import dan200.computercraft.api.lua.LuaException;
|
|
import dan200.computercraft.api.lua.LuaFunction;
|
|
import dan200.computercraft.api.peripheral.IPeripheral;
|
|
import dan200.computercraft.core.terminal.Terminal;
|
|
import dan200.computercraft.shared.util.StringUtil;
|
|
|
|
import javax.annotation.Nonnull;
|
|
import java.util.Optional;
|
|
|
|
/**
|
|
* The printer peripheral allows pages and books to be printed.
|
|
*
|
|
* @cc.module printer
|
|
*/
|
|
public class PrinterPeripheral implements IPeripheral
|
|
{
|
|
private final TilePrinter printer;
|
|
|
|
public PrinterPeripheral( TilePrinter printer )
|
|
{
|
|
this.printer = printer;
|
|
}
|
|
|
|
@Nonnull
|
|
@Override
|
|
public String getType()
|
|
{
|
|
return "printer";
|
|
}
|
|
|
|
// FIXME: There's a theoretical race condition here between getCurrentPage and then using the page. Ideally
|
|
// we'd lock on the page, consume it, and unlock.
|
|
|
|
// FIXME: None of our page modification functions actually mark the tile as dirty, so the page may not be
|
|
// persisted correctly.
|
|
|
|
@LuaFunction
|
|
public final void write( IArguments arguments ) throws LuaException
|
|
{
|
|
String text = StringUtil.toString( arguments.get( 0 ) );
|
|
Terminal page = getCurrentPage();
|
|
page.write( text );
|
|
page.setCursorPos( page.getCursorX() + text.length(), page.getCursorY() );
|
|
}
|
|
|
|
@LuaFunction
|
|
public final Object[] getCursorPos() throws LuaException
|
|
{
|
|
Terminal page = getCurrentPage();
|
|
int x = page.getCursorX();
|
|
int y = page.getCursorY();
|
|
return new Object[] { x + 1, y + 1 };
|
|
}
|
|
|
|
@LuaFunction
|
|
public final void setCursorPos( int x, int y ) throws LuaException
|
|
{
|
|
Terminal page = getCurrentPage();
|
|
page.setCursorPos( x - 1, y - 1 );
|
|
}
|
|
|
|
@LuaFunction
|
|
public final Object[] getPageSize() throws LuaException
|
|
{
|
|
Terminal page = getCurrentPage();
|
|
int width = page.getWidth();
|
|
int height = page.getHeight();
|
|
return new Object[] { width, height };
|
|
}
|
|
|
|
@LuaFunction( mainThread = true )
|
|
public final boolean newPage()
|
|
{
|
|
return printer.startNewPage();
|
|
}
|
|
|
|
@LuaFunction( mainThread = true )
|
|
public final boolean endPage() throws LuaException
|
|
{
|
|
getCurrentPage();
|
|
return printer.endCurrentPage();
|
|
}
|
|
|
|
@LuaFunction
|
|
public final void setPageTitle( Optional<String> title ) throws LuaException
|
|
{
|
|
getCurrentPage();
|
|
printer.setPageTitle( StringUtil.normaliseLabel( title.orElse( "" ) ) );
|
|
}
|
|
|
|
@LuaFunction
|
|
public final int getInkLevel()
|
|
{
|
|
return printer.getInkLevel();
|
|
}
|
|
|
|
@LuaFunction
|
|
public final int getPaperLevel()
|
|
{
|
|
return printer.getPaperLevel();
|
|
}
|
|
|
|
@Override
|
|
public boolean equals( IPeripheral other )
|
|
{
|
|
return other instanceof PrinterPeripheral && ((PrinterPeripheral) other).printer == printer;
|
|
}
|
|
|
|
@Nonnull
|
|
@Override
|
|
public Object getTarget()
|
|
{
|
|
return printer;
|
|
}
|
|
|
|
@Nonnull
|
|
private Terminal getCurrentPage() throws LuaException
|
|
{
|
|
Terminal currentPage = printer.getCurrentPage();
|
|
if( currentPage == null ) throw new LuaException( "Page not started" );
|
|
return currentPage;
|
|
}
|
|
}
|