mirror of
https://github.com/osmarks/mycorrhiza.git
synced 2024-12-11 21:10:26 +00:00
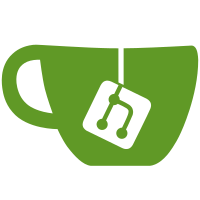
See https://mycorrhiza.lesarbr.es/hypha/configuration for an example. A copy of this example is stored at assets/config.ini. Use option -config-path to pass the config file. Note that all other CLI options have been removed. Some of them may be returned later. Also note that no real testing has been done.
104 lines
2.5 KiB
Go
104 lines
2.5 KiB
Go
package util
|
|
|
|
import (
|
|
"crypto/rand"
|
|
"encoding/hex"
|
|
"net/http"
|
|
"regexp"
|
|
"strings"
|
|
"unicode"
|
|
)
|
|
|
|
// TODO: make names match to fields of config file
|
|
var (
|
|
SiteName string
|
|
SiteNavIcon string
|
|
|
|
HomePage string
|
|
UserHypha string
|
|
HeaderLinksHypha string
|
|
|
|
ServerPort string
|
|
URL string
|
|
GeminiCertPath string
|
|
|
|
UseFixedAuth bool
|
|
FixedCredentialsPath string
|
|
|
|
WikiDir string
|
|
ConfigFilePath string
|
|
)
|
|
|
|
// LettersNumbersOnly keeps letters and numbers only in the given string.
|
|
func LettersNumbersOnly(s string) string {
|
|
var (
|
|
ret strings.Builder
|
|
usedUnderscore bool
|
|
)
|
|
for _, r := range s {
|
|
if unicode.IsLetter(r) || unicode.IsNumber(r) {
|
|
ret.WriteRune(r)
|
|
usedUnderscore = false
|
|
} else if !usedUnderscore {
|
|
ret.WriteRune('_')
|
|
usedUnderscore = true
|
|
}
|
|
}
|
|
return strings.Trim(ret.String(), "_")
|
|
}
|
|
|
|
// ShorterPath is used by handlerList to display shorter path to the files. It simply strips WikiDir.
|
|
func ShorterPath(path string) string {
|
|
if strings.HasPrefix(path, WikiDir) {
|
|
tmp := strings.TrimPrefix(path, WikiDir)
|
|
if tmp == "" {
|
|
return ""
|
|
}
|
|
return tmp[1:]
|
|
}
|
|
return path
|
|
}
|
|
|
|
// HTTP404Page writes a 404 error in the status, needed when no content is found on the page.
|
|
func HTTP404Page(w http.ResponseWriter, page string) {
|
|
w.Header().Set("Content-Type", "text/html;charset=utf-8")
|
|
w.WriteHeader(http.StatusNotFound)
|
|
w.Write([]byte(page))
|
|
}
|
|
|
|
// HTTP200Page wraps some frequently used things for successful 200 responses.
|
|
func HTTP200Page(w http.ResponseWriter, page string) {
|
|
w.Header().Set("Content-Type", "text/html;charset=utf-8")
|
|
w.WriteHeader(http.StatusOK)
|
|
w.Write([]byte(page))
|
|
}
|
|
|
|
func RandomString(n int) (string, error) {
|
|
bytes := make([]byte, n)
|
|
if _, err := rand.Read(bytes); err != nil {
|
|
return "", err
|
|
}
|
|
return hex.EncodeToString(bytes), nil
|
|
}
|
|
|
|
// Strip hypha name from all ancestor names, replace _ with spaces, title case
|
|
func BeautifulName(uglyName string) string {
|
|
if uglyName == "" {
|
|
return uglyName
|
|
}
|
|
return strings.Title(strings.ReplaceAll(uglyName, "_", " "))
|
|
}
|
|
|
|
// CanonicalName makes sure the `name` is canonical. A name is canonical if it is lowercase and all spaces are replaced with underscores.
|
|
func CanonicalName(name string) string {
|
|
return strings.ToLower(strings.ReplaceAll(name, " ", "_"))
|
|
}
|
|
|
|
// HyphaPattern is a pattern which all hyphae must match.
|
|
var HyphaPattern = regexp.MustCompile(`[^?!:#@><*|"\'&%{}]+`)
|
|
|
|
// IsCanonicalName checks if the `name` is canonical.
|
|
func IsCanonicalName(name string) bool {
|
|
return HyphaPattern.MatchString(name)
|
|
}
|