mirror of
https://github.com/osmarks/mycorrhiza.git
synced 2025-03-10 13:38:20 +00:00
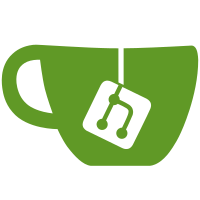
Didn't have the chance to migrate //all// templates just yet. We'll get there. * Implement yet another template system * Move orphans to the new system and fix a bug in it * Link orphans in the admin panel * Move the backlink handlers to the web package * Move auth routing to web * Move /user-list to the new system * Move change password and translate it * Move stuff * Move admin-related stuff to the web * Move a lot of files into internal dir Outside of it are web and stuff that needs further refactoring * Fix static not loading and de-qtpl tree * Move tree to internal * Keep the globe on the same line #230 * Revert "Keep the globe on the same line #230" This reverts commit ae78e5e459b1e980ba89bf29e61f75c0625ed2c7. * Migrate templates from hypview: delete, edit, start empty and existing WIP The delete media view was removed, I didn't even know it still existed as a GET. A rudiment. * Make views multi-file and break compilation * Megarefactoring of hypha views * Auth-related stuffs * Fix some of those weird imports * Migrate cat views * Fix cat js * Lower standards * Internalize trauma
119 lines
3.3 KiB
Go
119 lines
3.3 KiB
Go
package newtmpl
|
|
|
|
import (
|
|
"embed"
|
|
"fmt"
|
|
"github.com/bouncepaw/mycorrhiza/internal/cfg"
|
|
"github.com/bouncepaw/mycorrhiza/util"
|
|
"github.com/bouncepaw/mycorrhiza/web/viewutil"
|
|
"html/template"
|
|
"strings"
|
|
)
|
|
|
|
//go:embed *.html
|
|
var fs embed.FS
|
|
|
|
var base = template.Must(template.ParseFS(fs, "base.html"))
|
|
|
|
type Page struct {
|
|
TemplateEnglish *template.Template
|
|
TemplateRussian *template.Template
|
|
}
|
|
|
|
func NewPage(fs embed.FS, russianTranslation map[string]string, tmpls ...string) *Page {
|
|
must := template.Must
|
|
en := must(must(must(
|
|
base.Clone()).
|
|
Funcs(template.FuncMap{
|
|
"beautifulName": util.BeautifulName,
|
|
"inc": func(i int) int { return i + 1 },
|
|
"base": func(hyphaName string) string {
|
|
parts := strings.Split(hyphaName, "/")
|
|
return parts[len(parts)-1]
|
|
},
|
|
"beautifulLink": func(hyphaName string) template.HTML {
|
|
return template.HTML(
|
|
fmt.Sprintf(
|
|
`<a href="/hypha/%s">%s</a>`, hyphaName, hyphaName))
|
|
},
|
|
}).
|
|
Parse(fmt.Sprintf(`
|
|
{{define "wiki name"}}%s{{end}}
|
|
{{define "user hypha"}}%s{{end}}
|
|
`, cfg.WikiName, cfg.UserHypha))).
|
|
ParseFS(fs, tmpls...))
|
|
|
|
if cfg.UseAuth {
|
|
en = must(en.Parse(`
|
|
{{define "auth"}}
|
|
<ul class="top-bar__auth auth-links">
|
|
<li class="auth-links__box auth-links__user-box">
|
|
{{if .Meta.U.Group | eq "anon" }}
|
|
<a href="/login" class="auth-links__link auth-links__login-link">
|
|
{{block "login" .}}Login{{end}}
|
|
</a>
|
|
{{else}}
|
|
<a href="/hypha/{{block "user hypha" .}}{{end}}/{{.Meta.U.Name}}" class="auth-links__link auth-links__user-link">
|
|
{{beautifulName .Meta.U.Name}}
|
|
</a>
|
|
{{end}}
|
|
</li>
|
|
{{block "registration" .}}{{end}}
|
|
</ul>
|
|
{{end}}
|
|
`))
|
|
}
|
|
if cfg.AllowRegistration {
|
|
must(en.Parse(`{{define "registration"}}
|
|
{{if .Meta.U.Group | eq "anon"}}
|
|
<li class="auth-links__box auth-links__register-box">
|
|
<a href="/register" class="auth-links__link auth-links__register-link">
|
|
{{block "register" .}}Register{{end}}
|
|
</a>
|
|
</li>
|
|
{{end}}
|
|
{{end}}`))
|
|
}
|
|
|
|
russianTranslation["search by title"] = "Поиск по названию"
|
|
russianTranslation["login"] = "Войти"
|
|
russianTranslation["register"] = "Регистрация"
|
|
russianTranslation["cancel"] = "Отмена"
|
|
russianTranslation["categories"] = "Категории"
|
|
russianTranslation["remove from category title"] = "Убрать гифу из этой категории"
|
|
russianTranslation["placeholder"] = "Название категории..."
|
|
russianTranslation["add to category title"] = "Добавить гифу в эту категорию"
|
|
|
|
return &Page{
|
|
TemplateEnglish: en,
|
|
TemplateRussian: must(must(en.Clone()).
|
|
Parse(translationsIntoTemplates(russianTranslation))),
|
|
}
|
|
}
|
|
|
|
func translationsIntoTemplates(m map[string]string) string {
|
|
var sb strings.Builder
|
|
for k, v := range m {
|
|
sb.WriteString(fmt.Sprintf(`{{define "%s"}}%s{{end}}
|
|
`, k, v))
|
|
}
|
|
return sb.String()
|
|
}
|
|
|
|
func (p *Page) RenderTo(meta viewutil.Meta, data map[string]any) error {
|
|
data["Meta"] = meta
|
|
data["HeadElements"] = meta.HeadElements
|
|
data["BodyAttributes"] = meta.BodyAttributes
|
|
data["CommonScripts"] = cfg.CommonScripts
|
|
data["EditScripts"] = cfg.EditScripts
|
|
data["HeaderLinks"] = viewutil.HeaderLinks
|
|
data["UseAuth"] = cfg.UseAuth
|
|
|
|
tmpl := p.TemplateEnglish
|
|
if meta.LocaleIsRussian() {
|
|
tmpl = p.TemplateRussian
|
|
}
|
|
|
|
return tmpl.ExecuteTemplate(meta.W, "page", data)
|
|
}
|