mirror of
https://github.com/gnss-sdr/gnss-sdr
synced 2025-07-02 18:12:52 +00:00
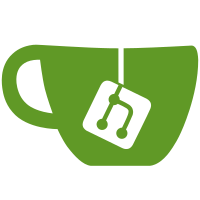
- The executable file and the default configuration file is now changed from "./install/mercurio" and "./conf/mercurio.conf" to "./install/gnss-sdr" and "./conf/gnss-sdr.conf", respectively. - Configuration file structure changed to define in a single entry the internal sampling frequency (after the signal conditioner). NOTICE that this change affects the all the adapters (acquisition, tracking, telemetry_decoder, observables, and PVT). All the adapters are now modified to work with this feature. - Moved several in-line GPS L1 CA parameters (a.k.a magic numbers..) to ./src/core/system_parameters/GPS_L1_CA.h definition file. - Tracking blocks now uses DOUBLE values in their outputs. - Observables and PVT now are separated. PVT and their associated libraries are moved to ./src/algorithms/PVT - Temporarily disabled the RINEX output (I am working on that!) - GNSS-SDR screen output now gives extended debug information of the receiver status and events. In the future, this output will be redirected to a log file. - Bug fixes: - FILE_SIGNAL_SOURCE now works correctly when the user configures GNSS-SDR to process the entire file. - GPS_L1_CA_DLL_PLL now computes correctly the PRN start values. - GPS_L1_CA_DLL_FLL_PLL now computes correctly the PRN start values. - Several modifications in GPS_L1_CA_Telemetry_Decoder, GPS_L1_CA_Observables, and GPS_L1_CA_PVT modules to fix the GPS position computation. - New features - Tracking blocks perform a signal integrity check against NaN outliers before the correlation process. - Tracking and PVT binary dump options are now documented and we provide MATLAB libraries and sample files to read it. Available in ./utils/matlab" and "./utils/matlab/libs" - Observables output rate can be configured. This option enables the GPS L1 CA PVT computation at a maximum rate of 1ms. - GPS_L1_CA_PVT now can perform a moving average Latitude, Longitude, and Altitude output for each of the Observables output. It is configurable using the configuration file. - Added Google Earth compatible Keyhole Markup Language (KML) output writer class (./src/algorithms/PVT/libs/kml_printer.h and ./src/algorithms/PVT/libs/kml_printer.cc ). You can see the receiver position directly using Google Earth. - We provide a master configuration file which includes an in-line documentation with all the new (and old) options. It can be found in ./conf/master.conf git-svn-id: https://svn.code.sf.net/p/gnss-sdr/code/trunk@84 64b25241-fba3-4117-9849-534c7e92360d
127 lines
3.6 KiB
C++
127 lines
3.6 KiB
C++
/*!
|
|
* \file kml_printer.cc
|
|
* \brief Prints PVT information to a GoogleEarth kml file
|
|
* \author Javier Arribas, 2011. jarribas(at)cttc.es
|
|
*
|
|
*
|
|
* -------------------------------------------------------------------------
|
|
*
|
|
* Copyright (C) 2010-2011 (see AUTHORS file for a list of contributors)
|
|
*
|
|
* GNSS-SDR is a software defined Global Navigation
|
|
* Satellite Systems receiver
|
|
*
|
|
* This file is part of GNSS-SDR.
|
|
*
|
|
* GNSS-SDR is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* at your option) any later version.
|
|
*
|
|
* GNSS-SDR is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with GNSS-SDR. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
* -------------------------------------------------------------------------
|
|
*/
|
|
|
|
#include "kml_printer.h"
|
|
#include <glog/log_severity.h>
|
|
#include <glog/logging.h>
|
|
|
|
#include <time.h>
|
|
|
|
bool kml_printer::set_headers(std::string filename)
|
|
{
|
|
time_t rawtime;
|
|
struct tm * timeinfo;
|
|
|
|
time ( &rawtime );
|
|
timeinfo = localtime ( &rawtime );
|
|
|
|
kml_file.open(filename.c_str());
|
|
if (kml_file.is_open())
|
|
{
|
|
DLOG(INFO)<<"KML printer writting on "<<filename.c_str();
|
|
kml_file<<"<?xml version=\"1.0\" encoding=\"UTF-8\"?>\r\n"
|
|
<<"<kml xmlns=\"http://www.opengis.net/kml/2.2\">\r\n"
|
|
<<" <Document>\r\n"
|
|
<<" <name>GNSS Track</name>\r\n"
|
|
<<" <description>GNSS-SDR Receiver position log file created at "<<asctime (timeinfo)
|
|
<<" </description>\r\n"
|
|
<<"<Style id=\"yellowLineGreenPoly\">\r\n"
|
|
<<" <LineStyle>\r\n"
|
|
<<" <color>7f00ffff</color>\r\n"
|
|
<<" <width>1</width>\r\n"
|
|
<<" </LineStyle>\r\n"
|
|
<<"<PolyStyle>\r\n"
|
|
<<" <color>7f00ff00</color>\r\n"
|
|
<<"</PolyStyle>\r\n"
|
|
<<"</Style>\r\n"
|
|
<<"<Placemark>\r\n"
|
|
<<"<name>GNSS-SDR PVT</name>\r\n"
|
|
<<"<description>GNSS-SDR position log</description>\r\n"
|
|
<<"<styleUrl>#yellowLineGreenPoly</styleUrl>\r\n"
|
|
<<"<LineString>\r\n"
|
|
<<"<extrude>0</extrude>\r\n"
|
|
<<"<tessellate>1</tessellate>\r\n"
|
|
<<"<altitudeMode>absolute</altitudeMode>\r\n"
|
|
<<"<coordinates>\r\n";
|
|
return true;
|
|
}else{
|
|
return false;
|
|
}
|
|
}
|
|
|
|
|
|
bool kml_printer::print_position(gps_l1_ca_ls_pvt* position,bool print_average_values)
|
|
{
|
|
double latitude;
|
|
double longitude;
|
|
double height;
|
|
if (print_average_values==false)
|
|
{
|
|
latitude=position->d_latitude_d;
|
|
longitude=position->d_longitude_d;
|
|
height=position->d_height_m;
|
|
}else{
|
|
latitude=position->d_avg_latitude_d;
|
|
longitude=position->d_avg_longitude_d;
|
|
height=position->d_avg_height_m;
|
|
}
|
|
if (kml_file.is_open())
|
|
{
|
|
kml_file<<longitude<<","<<latitude<<","<<height<<"\r\n";
|
|
return true;
|
|
}else
|
|
{
|
|
return false;
|
|
}
|
|
}
|
|
bool kml_printer::close_file()
|
|
{
|
|
if (kml_file.is_open())
|
|
{
|
|
kml_file<<"</coordinates>\r\n"
|
|
<<"</LineString>\r\n"
|
|
<<"</Placemark>\r\n"
|
|
<<"</Document>\r\n"
|
|
<<"</kml>";
|
|
kml_file.close();
|
|
return true;
|
|
}else{
|
|
return false;
|
|
}
|
|
}
|
|
kml_printer::kml_printer ()
|
|
{
|
|
}
|
|
|
|
kml_printer::~kml_printer ()
|
|
{
|
|
}
|