mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2025-07-07 04:22:50 +00:00
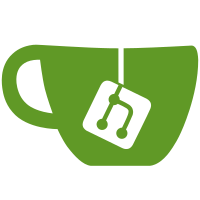
Without modified dates, new imports do not appear in recent file list. The equivalent of modified dates in ENEX is the 'updated' tag, with a date format similar to that of TW with the exception that the date format consists of a date stamp, a letter T, and a time stamp followed by Z for UTC presumably. There is no millisecond indicator. Not all ENEX notes have an update tag. The solution here is to apply creation date by default and then apply the update date if it is available. Dates are converted by stripping out Z and T and appending '000'.
77 lines
2.5 KiB
JavaScript
77 lines
2.5 KiB
JavaScript
/*\
|
|
title: $:/plugins/tiddlywiki/evernote/modules/enex-deserializer.js
|
|
type: application/javascript
|
|
module-type: tiddlerdeserializer
|
|
|
|
ENEX file deserializer
|
|
|
|
For details see: https://blog.evernote.com/tech/2013/08/08/evernote-export-format-enex/
|
|
|
|
\*/
|
|
(function(){
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
|
|
// DOMParser = require("$:/plugins/tiddlywiki/xmldom/dom-parser").DOMParser;
|
|
|
|
/*
|
|
Parse an ENEX file into tiddlers
|
|
*/
|
|
exports["application/enex+xml"] = function(text,fields) {
|
|
// Collect output tiddlers in an array
|
|
var results = [];
|
|
// Parse the XML document
|
|
var parser = new DOMParser(),
|
|
doc = parser.parseFromString(text,"application/xml");
|
|
// Output a report tiddler with information about the import
|
|
var enex = doc.querySelector("en-export");
|
|
results.push({
|
|
title: "Evernote Import Report",
|
|
text: "Evernote file imported on " + enex.getAttribute("export-date") + " from " + enex.getAttribute("application") + " (" + enex.getAttribute("version") + ")"
|
|
})
|
|
// Get all the "note" nodes
|
|
var noteNodes = doc.querySelectorAll("note");
|
|
$tw.utils.each(noteNodes,function(noteNode) {
|
|
var result = {
|
|
title: getTextContent(noteNode,"title"),
|
|
type: "text/html",
|
|
tags: [],
|
|
text: getTextContent(noteNode,"content"),
|
|
modified: getTextContent(noteNode,"created").replace("T","").replace("Z",""),
|
|
created: getTextContent(noteNode,"created").replace("T","").replace("Z","")
|
|
|
|
};
|
|
$tw.utils.each(noteNode.querySelectorAll("tag"),function(tagNode) {
|
|
result.tags.push(tagNode.textContent);
|
|
});
|
|
// If there's an update date, set modifiy date accordingly
|
|
$tw.utils.each(noteNode.querySelectorAll("updated"),function(modifiedNode) {
|
|
result["modified"] = modifiedNode.textContent.replace("T","").replace("Z","") + "000" ;
|
|
});
|
|
|
|
$tw.utils.each(noteNode.querySelectorAll("note-attributes"),function(attrNode) {
|
|
result[attrNode.tagName] = attrNode.textContent;
|
|
});
|
|
results.push(result);
|
|
$tw.utils.each(noteNode.querySelectorAll("resources"),function(resourceNode) {
|
|
results.push({
|
|
title: getTextContent(resourceNode,"resource-attributes>file-name"),
|
|
type: getTextContent(resourceNode,"mime"),
|
|
width: getTextContent(resourceNode,"width"),
|
|
height: getTextContent(resourceNode,"height"),
|
|
text: getTextContent(resourceNode,"data")
|
|
});
|
|
});
|
|
});
|
|
// Return the output tiddlers
|
|
return results;
|
|
};
|
|
|
|
function getTextContent(node,selector) {
|
|
return (node.querySelector(selector) || {}).textContent;
|
|
}
|
|
|
|
})();
|