mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2024-11-01 07:36:18 +00:00
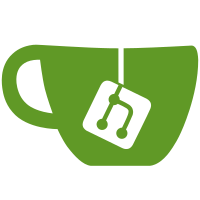
* Add support for JSON-formatted tiddler store, and make it the default The change to `getTiddlersAsJson()` is to allow experimentation * Move JSON tiddlers into their own store area, and fix support for encrypted tiddlers Also add a dummy old-style store area for backwards compatibility The current arrangement is that JSON tiddlers will always override old-style tiddlers. * Use the deserialiser mechanism to decode the content * Refactor $:/core/modules/deserializers.js before we start extending it Cleaning up the helper function names and ordering * Drop support for the "systemArea" div It was only used in really old v5.0.x * Update deserializer to support JSON store format and add some tests * Life UI restrictions on characters in fieldnames * Add another test case * Correct mis-merge * Remove toLowerCase() methods applied to fieldnames * Insert line breaks in output of getTiddlersAsJson (#5786) Rather than have the entire store on one line, insert a line break after each tiddler. * Refactor #5786 for backwards compatibility * Only read .tiddlywiki-tiddler-store blocks from script tags Prompted by @simonbaird's comment here: https://github.com/Jermolene/TiddlyWiki5/pull/5708#discussion_r648833367 * Clean up escaping of unsafe script characters It seems that escaping `<` is sufficient * Add docs from @saqimtiaz Thanks @saqimtiaz * Docs tweaks * Remove excess whitespace Thanks @simonbaird * Fix templates for lazy loading * Remove obsolete item from release note * Clean up whitespace * Docs for the jsontiddler widget * Fix whitespace Fixes #5840 * Comments * Fix newlines in JSON store area * Remove obsolete docs change Co-authored-by: Simon Baird <simon.baird@gmail.com>
135 lines
3.8 KiB
JavaScript
135 lines
3.8 KiB
JavaScript
/*\
|
|
title: $:/core/modules/widgets/fieldmangler.js
|
|
type: application/javascript
|
|
module-type: widget
|
|
|
|
Field mangler widget
|
|
|
|
\*/
|
|
(function(){
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
|
|
var Widget = require("$:/core/modules/widgets/widget.js").widget;
|
|
|
|
var FieldManglerWidget = function(parseTreeNode,options) {
|
|
this.initialise(parseTreeNode,options);
|
|
};
|
|
|
|
/*
|
|
Inherit from the base widget class
|
|
*/
|
|
FieldManglerWidget.prototype = new Widget();
|
|
|
|
/*
|
|
Render this widget into the DOM
|
|
*/
|
|
FieldManglerWidget.prototype.render = function(parent,nextSibling) {
|
|
this.addEventListeners([
|
|
{type: "tm-remove-field", handler: "handleRemoveFieldEvent"},
|
|
{type: "tm-add-field", handler: "handleAddFieldEvent"},
|
|
{type: "tm-remove-tag", handler: "handleRemoveTagEvent"},
|
|
{type: "tm-add-tag", handler: "handleAddTagEvent"}
|
|
]);
|
|
this.parentDomNode = parent;
|
|
this.computeAttributes();
|
|
this.execute();
|
|
this.renderChildren(parent,nextSibling);
|
|
};
|
|
|
|
/*
|
|
Compute the internal state of the widget
|
|
*/
|
|
FieldManglerWidget.prototype.execute = function() {
|
|
// Get our parameters
|
|
this.mangleTitle = this.getAttribute("tiddler",this.getVariable("currentTiddler"));
|
|
// Construct the child widgets
|
|
this.makeChildWidgets();
|
|
};
|
|
|
|
/*
|
|
Selectively refreshes the widget if needed. Returns true if the widget or any of its children needed re-rendering
|
|
*/
|
|
FieldManglerWidget.prototype.refresh = function(changedTiddlers) {
|
|
var changedAttributes = this.computeAttributes();
|
|
if(changedAttributes.tiddler) {
|
|
this.refreshSelf();
|
|
return true;
|
|
} else {
|
|
return this.refreshChildren(changedTiddlers);
|
|
}
|
|
};
|
|
|
|
FieldManglerWidget.prototype.handleRemoveFieldEvent = function(event) {
|
|
var tiddler = this.wiki.getTiddler(this.mangleTitle),
|
|
deletion = {};
|
|
deletion[event.param] = undefined;
|
|
this.wiki.addTiddler(new $tw.Tiddler(tiddler,deletion));
|
|
return false;
|
|
};
|
|
|
|
FieldManglerWidget.prototype.handleAddFieldEvent = function(event) {
|
|
var tiddler = this.wiki.getTiddler(this.mangleTitle),
|
|
addition = this.wiki.getModificationFields(),
|
|
addField = function(name,value) {
|
|
var trimmedName = name.trim();
|
|
if(!value && tiddler) {
|
|
value = tiddler.fields[trimmedName];
|
|
}
|
|
addition[trimmedName] = value || "";
|
|
return;
|
|
};
|
|
addition.title = this.mangleTitle;
|
|
if(typeof event.param === "string") {
|
|
addField(event.param,"");
|
|
}
|
|
if(typeof event.paramObject === "object") {
|
|
for(var name in event.paramObject) {
|
|
addField(name,event.paramObject[name]);
|
|
}
|
|
}
|
|
this.wiki.addTiddler(new $tw.Tiddler(tiddler,addition));
|
|
return false;
|
|
};
|
|
|
|
FieldManglerWidget.prototype.handleRemoveTagEvent = function(event) {
|
|
var tiddler = this.wiki.getTiddler(this.mangleTitle),
|
|
modification = this.wiki.getModificationFields();
|
|
if(tiddler && tiddler.fields.tags) {
|
|
var p = tiddler.fields.tags.indexOf(event.param);
|
|
if(p !== -1) {
|
|
modification.tags = (tiddler.fields.tags || []).slice(0);
|
|
modification.tags.splice(p,1);
|
|
if(modification.tags.length === 0) {
|
|
modification.tags = undefined;
|
|
}
|
|
this.wiki.addTiddler(new $tw.Tiddler(tiddler,modification));
|
|
}
|
|
}
|
|
return false;
|
|
};
|
|
|
|
FieldManglerWidget.prototype.handleAddTagEvent = function(event) {
|
|
var tiddler = this.wiki.getTiddler(this.mangleTitle),
|
|
modification = this.wiki.getModificationFields();
|
|
if(tiddler && typeof event.param === "string") {
|
|
var tag = event.param.trim();
|
|
if(tag !== "") {
|
|
modification.tags = (tiddler.fields.tags || []).slice(0);
|
|
$tw.utils.pushTop(modification.tags,tag);
|
|
this.wiki.addTiddler(new $tw.Tiddler(tiddler,modification));
|
|
}
|
|
} else if(typeof event.param === "string" && event.param.trim() !== "" && this.mangleTitle.trim() !== "") {
|
|
var tag = [];
|
|
tag.push(event.param.trim());
|
|
this.wiki.addTiddler(new $tw.Tiddler({title: this.mangleTitle, tags: tag},modification));
|
|
}
|
|
return false;
|
|
};
|
|
|
|
exports.fieldmangler = FieldManglerWidget;
|
|
|
|
})();
|