mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2024-11-06 01:56:20 +00:00
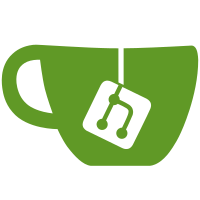
Get rid of the separate renderContext stack and instead have a parent pointer on renderer nodes. This lets us walk back up the render tree to resolve context references
51 lines
1.1 KiB
JavaScript
51 lines
1.1 KiB
JavaScript
/*\
|
|
title: $:/core/modules/widget/info.js
|
|
type: application/javascript
|
|
module-type: widget
|
|
|
|
Implements the info widget that displays various information about a specified tiddler.
|
|
|
|
\*/
|
|
(function(){
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
|
|
var InfoWidget = function(renderer) {
|
|
// Save state
|
|
this.renderer = renderer;
|
|
// Generate child nodes
|
|
this.generate();
|
|
};
|
|
|
|
InfoWidget.types = {
|
|
changecount: function(options) {return options.wiki.getChangeCount(options.title);}
|
|
};
|
|
|
|
InfoWidget.prototype.generate = function() {
|
|
// Get attributes
|
|
this.tiddlerTitle = this.renderer.getAttribute("tiddler",this.tiddlerTitle);
|
|
this.type = this.renderer.getAttribute("type","changecount");
|
|
// Get the appropriate value for the current tiddler
|
|
var value = "",
|
|
fn = InfoWidget.types[this.type];
|
|
if(fn) {
|
|
value = fn({
|
|
wiki: this.renderer.renderTree.wiki,
|
|
title: this.tiddlerTitle
|
|
});
|
|
}
|
|
// Set the element
|
|
this.tag = "span";
|
|
this.attributes = {};
|
|
this.children = this.renderer.renderTree.createRenderers(this.renderer,[{
|
|
type: "text",
|
|
text: value
|
|
}]);
|
|
};
|
|
|
|
exports.info = InfoWidget;
|
|
|
|
})();
|