mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2024-11-08 19:09:57 +00:00
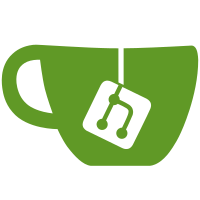
Fixes #717 The issue was that under Windows we generate text nodes that contained CRLF as a linebreak (rather than just LF as usual). The subtle problem is that when these strings are placed in the DOM via createTextNode(), the CR character is treated as a printable character, not whitespace. When creating DOM notes with innerHTML or as part of a static HTML document the HTML parser will strip out the CR characters. The hacky solution is to manually remove CRs before building the text node.
63 lines
1.3 KiB
JavaScript
Executable File
63 lines
1.3 KiB
JavaScript
Executable File
/*\
|
|
title: $:/core/modules/widgets/text.js
|
|
type: application/javascript
|
|
module-type: widget
|
|
|
|
Text node widget
|
|
|
|
\*/
|
|
(function(){
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
|
|
var Widget = require("$:/core/modules/widgets/widget.js").widget;
|
|
|
|
var TextNodeWidget = function(parseTreeNode,options) {
|
|
this.initialise(parseTreeNode,options);
|
|
};
|
|
|
|
/*
|
|
Inherit from the base widget class
|
|
*/
|
|
TextNodeWidget.prototype = new Widget();
|
|
|
|
/*
|
|
Render this widget into the DOM
|
|
*/
|
|
TextNodeWidget.prototype.render = function(parent,nextSibling) {
|
|
this.parentDomNode = parent;
|
|
this.computeAttributes();
|
|
this.execute();
|
|
var text = this.getAttribute("text",this.parseTreeNode.text);
|
|
text = text.replace(/\r/mg,"");
|
|
var textNode = this.document.createTextNode(text);
|
|
parent.insertBefore(textNode,nextSibling);
|
|
this.domNodes.push(textNode);
|
|
};
|
|
|
|
/*
|
|
Compute the internal state of the widget
|
|
*/
|
|
TextNodeWidget.prototype.execute = function() {
|
|
// Nothing to do for a text node
|
|
};
|
|
|
|
/*
|
|
Selectively refreshes the widget if needed. Returns true if the widget or any of its children needed re-rendering
|
|
*/
|
|
TextNodeWidget.prototype.refresh = function(changedTiddlers) {
|
|
var changedAttributes = this.computeAttributes();
|
|
if(changedAttributes.text) {
|
|
this.refreshSelf();
|
|
return true;
|
|
} else {
|
|
return false;
|
|
}
|
|
};
|
|
|
|
exports.text = TextNodeWidget;
|
|
|
|
})();
|