mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2024-07-18 18:04:28 +00:00
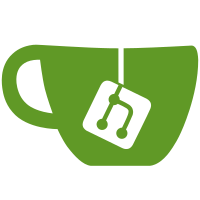
There are still some whitespace and attribute ordering issues, but the result runs correctly.
70 lines
1.8 KiB
JavaScript
Executable File
70 lines
1.8 KiB
JavaScript
Executable File
//--
|
|
//-- Wizard support
|
|
//--
|
|
|
|
function Wizard(elem)
|
|
{
|
|
if(elem) {
|
|
this.formElem = findRelated(elem,"wizard","className");
|
|
this.bodyElem = findRelated(this.formElem.firstChild,"wizardBody","className","nextSibling");
|
|
this.footElem = findRelated(this.formElem.firstChild,"wizardFooter","className","nextSibling");
|
|
} else {
|
|
this.formElem = null;
|
|
this.bodyElem = null;
|
|
this.footElem = null;
|
|
}
|
|
}
|
|
|
|
Wizard.prototype.setValue = function(name,value)
|
|
{
|
|
jQuery(this.formElem).data(name, value);
|
|
};
|
|
|
|
Wizard.prototype.getValue = function(name)
|
|
{
|
|
return this.formElem ? jQuery(this.formElem).data(name) : null;
|
|
};
|
|
|
|
Wizard.prototype.createWizard = function(place,title)
|
|
{
|
|
this.formElem = createTiddlyElement(place,"form",null,"wizard");
|
|
createTiddlyElement(this.formElem,"h1",null,null,title);
|
|
this.bodyElem = createTiddlyElement(this.formElem,"div",null,"wizardBody");
|
|
this.footElem = createTiddlyElement(this.formElem,"div",null,"wizardFooter");
|
|
return this.formElem;
|
|
};
|
|
|
|
Wizard.prototype.clear = function()
|
|
{
|
|
jQuery(this.bodyElem).empty();
|
|
};
|
|
|
|
Wizard.prototype.setButtons = function(buttonInfo,status)
|
|
{
|
|
jQuery(this.footElem).empty();
|
|
var t;
|
|
for(t=0; t<buttonInfo.length; t++) {
|
|
createTiddlyButton(this.footElem,buttonInfo[t].caption,buttonInfo[t].tooltip,buttonInfo[t].onClick);
|
|
insertSpacer(this.footElem);
|
|
}
|
|
if(typeof status == "string") {
|
|
createTiddlyElement(this.footElem,"span",null,"status",status);
|
|
}
|
|
};
|
|
|
|
Wizard.prototype.addStep = function(stepTitle,html)
|
|
{
|
|
jQuery(this.bodyElem).empty();
|
|
var w = createTiddlyElement(this.bodyElem,"div");
|
|
createTiddlyElement(w,"h2",null,null,stepTitle);
|
|
var step = createTiddlyElement(w,"div",null,"wizardStep");
|
|
step.innerHTML = html;
|
|
applyHtmlMacros(step,tiddler);
|
|
};
|
|
|
|
Wizard.prototype.getElement = function(name)
|
|
{
|
|
return this.formElem.elements[name];
|
|
};
|
|
|