mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2024-06-25 23:03:15 +00:00
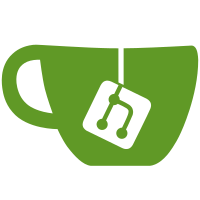
* Implement insertafter operator (like insertbefore) Currently, the behavior of insertafter if the target is not found is to append the inserted tiddler to the end of the list, like insertbefore does. In the next commit, we'll add a suffix to customize what both insertafter and insertbefore do when the target is not found. * Add failing tests for insertafter suffixes Also includes tests for insertbefore suffixes (start/end), since we'll be implementing both of those at the same time. * Add start/end suffixes for insertafter/before The tests that exercise the start/end suffixes now pass.
47 lines
1.1 KiB
JavaScript
47 lines
1.1 KiB
JavaScript
/*\
|
|
title: $:/core/modules/filters/insertbefore.js
|
|
type: application/javascript
|
|
module-type: filteroperator
|
|
|
|
Insert an item before another item in a list
|
|
|
|
\*/
|
|
(function(){
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
|
|
/*
|
|
Order a list
|
|
*/
|
|
exports.insertbefore = function(source,operator,options) {
|
|
var results = [];
|
|
source(function(tiddler,title) {
|
|
results.push(title);
|
|
});
|
|
var target = operator.operands[1] || (options.widget && options.widget.getVariable(operator.suffix || "currentTiddler"));
|
|
if(target !== operator.operand) {
|
|
// Remove the entry from the list if it is present
|
|
var pos = results.indexOf(operator.operand);
|
|
if(pos !== -1) {
|
|
results.splice(pos,1);
|
|
}
|
|
// Insert the entry before the target marker
|
|
pos = results.indexOf(target);
|
|
if(pos !== -1) {
|
|
results.splice(pos,0,operator.operand);
|
|
} else {
|
|
var suffix = operator.operands.length > 1 ? operator.suffix : "";
|
|
if(suffix == "start") {
|
|
results.splice(0,0,operator.operand);
|
|
} else {
|
|
results.push(operator.operand);
|
|
}
|
|
}
|
|
}
|
|
return results;
|
|
};
|
|
|
|
})();
|