mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2024-07-01 09:43:16 +00:00
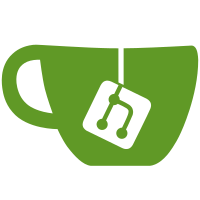
We do this so that we don’t accidentally modify shadow tiddlers when we drag them to reorder them within their tag parent. Otherwise, moving a toolbar button like $:/core/ui/Buttons/permaview in the control panel will override the shadow tiddler.
97 lines
2.9 KiB
JavaScript
97 lines
2.9 KiB
JavaScript
/*\
|
|
title: $:/core/modules/widgets/action-listops.js
|
|
type: application/javascript
|
|
module-type: widget
|
|
|
|
Action widget to apply list operations to any tiddler field (defaults to the 'list' field of the current tiddler)
|
|
|
|
\*/
|
|
(function() {
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
var Widget = require("$:/core/modules/widgets/widget.js").widget;
|
|
var ActionListopsWidget = function(parseTreeNode, options) {
|
|
this.initialise(parseTreeNode, options);
|
|
};
|
|
/**
|
|
* Inherit from the base widget class
|
|
*/
|
|
ActionListopsWidget.prototype = new Widget();
|
|
/**
|
|
* Render this widget into the DOM
|
|
*/
|
|
ActionListopsWidget.prototype.render = function(parent, nextSibling) {
|
|
this.computeAttributes();
|
|
this.execute();
|
|
};
|
|
/**
|
|
* Compute the internal state of the widget
|
|
*/
|
|
ActionListopsWidget.prototype.execute = function() {
|
|
// Get our parameters
|
|
this.target = this.getAttribute("$tiddler", this.getVariable(
|
|
"currentTiddler"));
|
|
this.filter = this.getAttribute("$filter");
|
|
this.subfilter = this.getAttribute("$subfilter");
|
|
this.listField = this.getAttribute("$field", "list");
|
|
this.listIndex = this.getAttribute("$index");
|
|
this.filtertags = this.getAttribute("$tags");
|
|
};
|
|
/**
|
|
* Refresh the widget by ensuring our attributes are up to date
|
|
*/
|
|
ActionListopsWidget.prototype.refresh = function(changedTiddlers) {
|
|
var changedAttributes = this.computeAttributes();
|
|
if(changedAttributes.$tiddler || changedAttributes.$filter ||
|
|
changedAttributes.$subfilter || changedAttributes.$field ||
|
|
changedAttributes.$index || changedAttributes.$tags) {
|
|
this.refreshSelf();
|
|
return true;
|
|
}
|
|
return this.refreshChildren(changedTiddlers);
|
|
};
|
|
/**
|
|
* Invoke the action associated with this widget
|
|
*/
|
|
ActionListopsWidget.prototype.invokeAction = function(triggeringWidget,
|
|
event) {
|
|
//Apply the specified filters to the lists
|
|
var field = this.listField,
|
|
index,
|
|
type = "!!",
|
|
list = this.listField;
|
|
if(this.listIndex) {
|
|
field = undefined;
|
|
index = this.listIndex;
|
|
type = "##";
|
|
list = this.listIndex;
|
|
}
|
|
if(this.filter) {
|
|
this.wiki.setText(this.target, field, index, $tw.utils.stringifyList(
|
|
this.wiki
|
|
.filterTiddlers(this.filter, this)));
|
|
}
|
|
if(this.subfilter) {
|
|
var subfilter = "[list[" + this.target + type + list + "]] " + this.subfilter;
|
|
this.wiki.setText(this.target, field, index, $tw.utils.stringifyList(
|
|
this.wiki
|
|
.filterTiddlers(subfilter, this)));
|
|
}
|
|
if(this.filtertags) {
|
|
var tiddler = this.wiki.getTiddler(this.target),
|
|
oldtags = tiddler ? (tiddler.fields.tags || []).slice(0) : [],
|
|
tagfilter = "[list[" + this.target + "!!tags]] " + this.filtertags,
|
|
newtags = this.wiki.filterTiddlers(tagfilter,this);
|
|
if($tw.utils.stringifyList(oldtags.sort()) !== $tw.utils.stringifyList(newtags.sort())) {
|
|
this.wiki.setText(this.target,"tags",undefined,$tw.utils.stringifyList(newtags));
|
|
}
|
|
}
|
|
return true; // Action was invoked
|
|
};
|
|
|
|
exports["action-listops"] = ActionListopsWidget;
|
|
|
|
})();
|