mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2024-11-04 17:16:18 +00:00
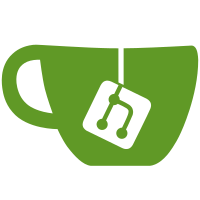
With this commit tag pills will now choose for the foreground colour either the current palette “foreground” or “background” colours, according to which has the higher contrast. It’s something @gernert has expressed an interest in in the past, and I’ve tended to agree that it is a nice piece of polish. It opens up the possibility of paler colours for tag pills than are currently possible. The trouble is that in order to implement it I’ve had to bring in a third party library for parsing CSS colours. It weighs in just over 9KB, making quite a lot of weight for such a small feature. I don’t see any other immediate uses for the colour parsing library either. So, I’m undecided at the moment whether this should stay in the core.
46 lines
1.2 KiB
JavaScript
46 lines
1.2 KiB
JavaScript
/*\
|
|
title: $:/core/modules/macros/contrastcolour.js
|
|
type: application/javascript
|
|
module-type: macro
|
|
|
|
Macro to choose which of two colours has the highest contrast with a base colour
|
|
|
|
\*/
|
|
(function(){
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
|
|
/*
|
|
Information about this macro
|
|
*/
|
|
|
|
exports.name = "contrastcolour";
|
|
|
|
exports.params = [
|
|
{name: "target"},
|
|
{name: "fallbackTarget"},
|
|
{name: "colourA"},
|
|
{name: "colourB"}
|
|
];
|
|
|
|
/*
|
|
Run the macro
|
|
*/
|
|
exports.run = function(target,fallbackTarget,colourA,colourB) {
|
|
var rgbTarget = $tw.utils.parseCSSColor(target) || $tw.utils.parseCSSColor(fallbackTarget);
|
|
if(!rgbTarget) {
|
|
return colourA;
|
|
}
|
|
// Colour brightness formula derived from http://www.w3.org/WAI/ER/WD-AERT/#color-contrast
|
|
var rgbColourA = $tw.utils.parseCSSColor(colourA),
|
|
rgbColourB = $tw.utils.parseCSSColor(colourB),
|
|
brightnessTarget = rgbTarget[0] * 0.299 + rgbTarget[1] * 0.587 + rgbTarget[2] * 0.114,
|
|
brightnessA = rgbColourA[0] * 0.299 + rgbColourA[1] * 0.587 + rgbColourA[2] * 0.114,
|
|
brightnessB = rgbColourB[0] * 0.299 + rgbColourB[1] * 0.587 + rgbColourB[2] * 0.114;
|
|
return Math.abs(brightnessTarget - brightnessA) > Math.abs(brightnessTarget - brightnessB) ? colourA : colourB;
|
|
};
|
|
|
|
})();
|