mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2024-10-31 23:26:18 +00:00
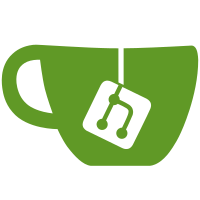
Passing an arbitrary variable allows us to e.g. reuse the export filters as shown in the example
57 lines
1.2 KiB
JavaScript
Executable File
57 lines
1.2 KiB
JavaScript
Executable File
/*\
|
|
title: $:/core/modules/commands/rendertiddler.js
|
|
type: application/javascript
|
|
module-type: command
|
|
|
|
Command to render a tiddler and save it to a file
|
|
|
|
\*/
|
|
(function(){
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
|
|
exports.info = {
|
|
name: "rendertiddler",
|
|
synchronous: false
|
|
};
|
|
|
|
var Command = function(params,commander,callback) {
|
|
this.params = params;
|
|
this.commander = commander;
|
|
this.callback = callback;
|
|
};
|
|
|
|
Command.prototype.execute = function() {
|
|
if(this.params.length < 2) {
|
|
return "Missing filename";
|
|
}
|
|
var self = this,
|
|
fs = require("fs"),
|
|
path = require("path"),
|
|
title = this.params[0],
|
|
filename = path.resolve(this.commander.outputPath,this.params[1]),
|
|
type = this.params[2] || "text/html",
|
|
template = this.params[3],
|
|
name = this.params[4],
|
|
value = this.params[5],
|
|
variables = {};
|
|
$tw.utils.createFileDirectories(filename);
|
|
if(template) {
|
|
variables.currentTiddler = title;
|
|
title = template;
|
|
}
|
|
if(name && value) {
|
|
variables[name] = value;
|
|
}
|
|
fs.writeFile(filename,this.commander.wiki.renderTiddler(type,title,{variables: variables}),"utf8",function(err) {
|
|
self.callback(err);
|
|
});
|
|
return null;
|
|
};
|
|
|
|
exports.Command = Command;
|
|
|
|
})();
|