mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2024-11-06 01:56:20 +00:00
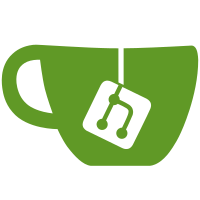
Get rid of the separate renderContext stack and instead have a parent pointer on renderer nodes. This lets us walk back up the render tree to resolve context references
59 lines
1.7 KiB
JavaScript
59 lines
1.7 KiB
JavaScript
/*\
|
|
title: $:/core/modules/widget/linkcatcher.js
|
|
type: application/javascript
|
|
module-type: widget
|
|
|
|
Implements the linkcatcher widget. It intercepts navigation events from its children, preventing normal navigation, and instead stores the name of the target tiddler in the text reference specified in the `to` attribute.
|
|
|
|
Using the linkcatcher widget allows the linking mechanism to be used for tasks like selecting the current theme tiddler from a list.
|
|
|
|
\*/
|
|
(function(){
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
|
|
var LinkCatcherWidget = function(renderer) {
|
|
// Save state
|
|
this.renderer = renderer;
|
|
// Generate child nodes
|
|
this.generate();
|
|
};
|
|
|
|
LinkCatcherWidget.prototype.generate = function() {
|
|
// Get our attributes
|
|
this.to = this.renderer.getAttribute("to");
|
|
// Set the element
|
|
this.tag = "div";
|
|
this.attributes = {
|
|
"class": "tw-linkcatcher"
|
|
};
|
|
this.children = this.renderer.renderTree.createRenderers(this.renderer,this.renderer.parseTreeNode.children);
|
|
this.events = [
|
|
{name: "tw-navigate", handlerObject: this, handlerMethod: "handleNavigateEvent"}
|
|
];
|
|
};
|
|
|
|
LinkCatcherWidget.prototype.refreshInDom = function(changedAttributes,changedTiddlers) {
|
|
// We don't need to refresh ourselves, so just refresh any child nodes
|
|
$tw.utils.each(this.children,function(node) {
|
|
if(node.refreshInDom) {
|
|
node.refreshInDom(changedTiddlers);
|
|
}
|
|
});
|
|
};
|
|
|
|
// Navigate to a specified tiddler
|
|
LinkCatcherWidget.prototype.handleNavigateEvent = function(event) {
|
|
if(this.to) {
|
|
this.renderer.renderTree.wiki.setTextReference(this.to,event.navigateTo,this.renderer.tiddlerTitle);
|
|
}
|
|
event.stopPropagation();
|
|
return false;
|
|
};
|
|
|
|
exports.linkcatcher = LinkCatcherWidget;
|
|
|
|
})();
|