mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2024-11-09 19:39:57 +00:00
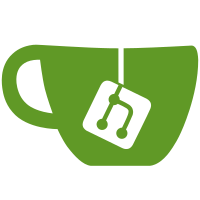
We now use a fake DOM implementation on the server to let us share more rendering code between the text output vs. DOM output paths.
55 lines
1.4 KiB
JavaScript
55 lines
1.4 KiB
JavaScript
/*\
|
|
title: $:/core/modules/commands/savetiddlers.js
|
|
type: application/javascript
|
|
module-type: command
|
|
|
|
Command to save several tiddlers to a file
|
|
|
|
\*/
|
|
(function(){
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
|
|
exports.info = {
|
|
name: "savetiddlers",
|
|
synchronous: false
|
|
};
|
|
|
|
var Command = function(params,commander,callback) {
|
|
this.params = params;
|
|
this.commander = commander;
|
|
this.callback = callback;
|
|
};
|
|
|
|
Command.prototype.execute = function() {
|
|
if(this.params.length < 2) {
|
|
return "Missing filename";
|
|
}
|
|
var self = this,
|
|
fs = require("fs"),
|
|
path = require("path"),
|
|
wiki = this.commander.wiki,
|
|
filter = this.params[0],
|
|
template = this.params[1],
|
|
pathname = this.params[2],
|
|
type = this.params[3] || "text/html",
|
|
extension = this.params[4] || ".html",
|
|
parser = wiki.parseTiddler(template),
|
|
tiddlers = wiki.filterTiddlers(filter);
|
|
$tw.utils.each(tiddlers,function(title) {
|
|
var renderTree = new $tw.WikiRenderTree(parser,{wiki: wiki, context: {tiddlerTitle: title}, document: $tw.document});
|
|
renderTree.execute();
|
|
var container = $tw.document.createElement("div");
|
|
renderTree.renderInDom(container);
|
|
var text = type === "text/html" ? container.innerHTML : container.textContent;
|
|
fs.writeFileSync(path.resolve(pathname,encodeURIComponent(title) + extension),text,"utf8");
|
|
});
|
|
return null;
|
|
};
|
|
|
|
exports.Command = Command;
|
|
|
|
})();
|