mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2024-11-27 03:57:21 +00:00
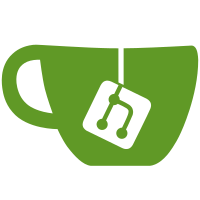
* mws authentication * add more tests and permission checkers * add logic to ensure that only authenticated users' requests are handled * add custom login page * Implement user authentication as well as session handling * work on user operations authorization * add middleware to route handlers for bags & tiddlers routes * add feature that only returns the tiddlers and bags which the user has permission to access on index page * refactor auth routes & added user management page * fix Ci Test failure issue * fix users list page, add manage roles page * add commands and scripts to create new user & assign roles and permissions * resolved ci-test failure * add ACL permissions to bags & tiddlers on creation * fix comments and access control list bug * fix indentation issues * working on user profile edit * remove list users command & added support for database in server options * implement user profile update and password change feature * update plugin readme * implement command which triggers protected mode on the server * revert server-wide auth flag. Implement selective authorization * ACL management feature * Complete Access control list implementation * Added support to manage users' assigned role by admin * fix comments * fix comment
66 lines
1.5 KiB
JavaScript
66 lines
1.5 KiB
JavaScript
/*\
|
|
title: $:/plugins/tiddlywiki/multiwikiclient/managetiddleraction.js
|
|
type: application/javascript
|
|
module-type: widget
|
|
|
|
A widget to manage tiddler actions.
|
|
\*/
|
|
(function(){
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
|
|
var Widget = require("$:/core/modules/widgets/widget.js").widget;
|
|
|
|
var ManageTiddlerAction = function(parseTreeNode,options) {
|
|
this.initialise(parseTreeNode,options);
|
|
};
|
|
|
|
/*
|
|
Inherit from the base widget class
|
|
*/
|
|
ManageTiddlerAction.prototype = new Widget();
|
|
|
|
/*
|
|
Render this widget into the DOM
|
|
*/
|
|
ManageTiddlerAction.prototype.render = function(parent,nextSibling) {
|
|
this.computeAttributes();
|
|
this.execute();
|
|
};
|
|
|
|
/*
|
|
Compute the internal state of the widget
|
|
*/
|
|
ManageTiddlerAction.prototype.execute = function() {
|
|
this.tiddler = this.getAttribute("tiddler");
|
|
};
|
|
|
|
/*
|
|
Invoke the action associated with this widget
|
|
*/
|
|
ManageTiddlerAction.prototype.invokeAction = function(triggeringWidget,event) {
|
|
var pathname = window.location.pathname;
|
|
var paths = pathname.split("/");
|
|
var recipeName = paths[paths.length - 1];
|
|
var bagName = document.querySelector("h1.tc-site-title").innerHTML;
|
|
window.location.href = "/admin/acl/"+recipeName+"/"+bagName;
|
|
};
|
|
|
|
/*
|
|
Refresh the widget by ensuring our attributes are up to date
|
|
*/
|
|
ManageTiddlerAction.prototype.refresh = function(changedTiddlers) {
|
|
var changedAttributes = this.computeAttributes();
|
|
if(changedAttributes.tiddler) {
|
|
this.refreshSelf();
|
|
return true;
|
|
}
|
|
return this.refreshChildren(changedTiddlers);
|
|
};
|
|
|
|
exports["action-managetiddler"] = ManageTiddlerAction;
|
|
|
|
})();
|