mirror of
https://github.com/Jermolene/TiddlyWiki5
synced 2025-07-04 11:02:51 +00:00
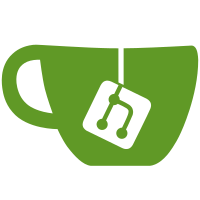
The objective is to add a $depth attribute so that it is possible to reach up to retrieve the parameters of ancestor transclusions. However, doing so requires changing the encoding of parameter names so that it is not possible for a user parameter to clash with an attribute like $depth. So now we have to double up dollars on any attribute names seen by the parameters widget, just like with the transclude widget itself.
100 lines
2.6 KiB
JavaScript
100 lines
2.6 KiB
JavaScript
/*\
|
|
title: $:/core/modules/widgets/parameters.js
|
|
type: application/javascript
|
|
module-type: widget
|
|
|
|
Widget for definition of transclusion parameters
|
|
|
|
\*/
|
|
(function(){
|
|
|
|
/*jslint node: true, browser: true */
|
|
/*global $tw: false */
|
|
"use strict";
|
|
|
|
var Widget = require("$:/core/modules/widgets/widget.js").widget,
|
|
TranscludeWidget = require("$:/core/modules/widgets/transclude.js").transclude;
|
|
|
|
var ParametersWidget = function(parseTreeNode,options) {
|
|
// Initialise
|
|
this.initialise(parseTreeNode,options);
|
|
};
|
|
|
|
/*
|
|
Inherit from the base widget class
|
|
*/
|
|
ParametersWidget.prototype = Object.create(Widget.prototype);
|
|
|
|
/*
|
|
Render this widget into the DOM
|
|
*/
|
|
ParametersWidget.prototype.render = function(parent,nextSibling) {
|
|
// Call the constructor
|
|
Widget.call(this);
|
|
this.parentDomNode = parent;
|
|
this.computeAttributes();
|
|
this.execute();
|
|
this.renderChildren(parent,nextSibling);
|
|
};
|
|
|
|
/*
|
|
Compute the internal state of the widget
|
|
*/
|
|
ParametersWidget.prototype.execute = function() {
|
|
var self = this;
|
|
this.parametersDepth = parseInt(this.getAttribute("$depth","1"),10) || 1;
|
|
// Find the parent transclusions
|
|
var pointer = this.parentWidget,
|
|
depth = this.parametersDepth;
|
|
while(pointer) {
|
|
if(pointer instanceof TranscludeWidget) {
|
|
depth--;
|
|
if(depth === 0) {
|
|
break;
|
|
}
|
|
}
|
|
pointer = pointer.parentWidget;
|
|
}
|
|
// Process each parameter
|
|
if(pointer instanceof TranscludeWidget) {
|
|
// Get the value for each defined parameter
|
|
$tw.utils.each($tw.utils.getOrderedAttributesFromParseTreeNode(self.parseTreeNode),function(attr,index) {
|
|
var name = attr.name;
|
|
// If the attribute name starts with $$ then reduce to a single dollar
|
|
if(name.substr(0,2) === "$$") {
|
|
name = name.substr(1);
|
|
}
|
|
var value = pointer.getTransclusionParameter(name,index,self.getAttribute(attr.name,""));
|
|
self.setVariable(name,value);
|
|
});
|
|
// Assign any metaparameters
|
|
var assignMetaParameter = function(name) {
|
|
var variableName = self.getAttribute("$" + name);
|
|
if(variableName !== undefined) {
|
|
self.setVariable(variableName,pointer.getTransclusionMetaParameter(name));
|
|
}
|
|
};
|
|
assignMetaParameter("parseAsInline");
|
|
assignMetaParameter("parseTreeNodes");
|
|
assignMetaParameter("params");
|
|
}
|
|
// Construct the child widgets
|
|
this.makeChildWidgets();
|
|
};
|
|
|
|
/*
|
|
Refresh the widget by ensuring our attributes are up to date
|
|
*/
|
|
ParametersWidget.prototype.refresh = function(changedTiddlers) {
|
|
var changedAttributes = this.computeAttributes();
|
|
if(Object.keys(changedAttributes).length) {
|
|
this.refreshSelf();
|
|
return true;
|
|
}
|
|
return this.refreshChildren(changedTiddlers);
|
|
};
|
|
|
|
exports.parameters = ParametersWidget;
|
|
|
|
})();
|