mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2025-07-05 11:32:53 +00:00
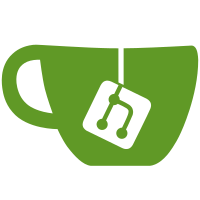
Look, I originally had this split into several commits, but lots of other cleanups got mixed in. I then backported some of the cleanups to 1.12, did other tidy ups there, and eventually the web of merges was unreadable. Yes, this is a horrible mess, but it's still nicer than it was. Anyway, changes: - Flatten everything. For instance, there are now three instances of BlockComputer, two BlockTurtle, ItemPocketComputer. There's also no more BlockPeripheral (thank heavens) - there's separate block classes for each peripheral type. - Remove pretty much all legacy code. As we're breaking world compatibility anyway, we can remove all the code to load worlds from 1.4 days. - The command system is largely rewriten to take advantage of 1.13's new system. It's very fancy! - WidgetTerminal now uses Minecraft's "GUI listener" system. - BREAKING CHANGE: All the codes in keys.lua are different, due to the move to LWJGL 3. Hopefully this won't have too much of an impact. I don't want to map to the old key codes on the Java side, as there always ends up being small but slight inconsistencies. IMO it's better to make a clean break - people should be using keys rather than hard coding the constants anyway. - commands.list now allows fetching sub-commands. The ROM has already been updated to allow fancy usage such as commands.time.set("noon"). - Turtles, modems and cables can be waterlogged.
44 lines
2.1 KiB
Java
44 lines
2.1 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2019. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
|
|
package dan200.computercraft.shared.command;
|
|
|
|
import com.mojang.brigadier.exceptions.Dynamic2CommandExceptionType;
|
|
import com.mojang.brigadier.exceptions.DynamicCommandExceptionType;
|
|
import com.mojang.brigadier.exceptions.SimpleCommandExceptionType;
|
|
import net.minecraft.util.text.TextComponentTranslation;
|
|
|
|
public final class Exceptions
|
|
{
|
|
public static final DynamicCommandExceptionType COMPUTER_ARG_NONE = translated1( "argument.computercraft.computer.no_matching" );
|
|
public static final Dynamic2CommandExceptionType COMPUTER_ARG_MANY = translated2( "argument.computercraft.computer.many_matching" );
|
|
|
|
public static final DynamicCommandExceptionType TRACKING_FIELD_ARG_NONE = translated1( "argument.computercraft.tacking_field.no_field" );
|
|
|
|
static final SimpleCommandExceptionType NOT_TRACKING_EXCEPTION = translated( "commands.computercraft.track.stop.not_enabled" );
|
|
static final SimpleCommandExceptionType NO_TIMINGS_EXCEPTION = translated( "commands.computercraft.track.dump.no_timings" );
|
|
|
|
static final SimpleCommandExceptionType TP_NOT_THERE = translated( "commands.computercraft.tp.not_there" );
|
|
static final SimpleCommandExceptionType TP_NOT_PLAYER = translated( "commands.computercraft.tp.not_player" );
|
|
|
|
public static final SimpleCommandExceptionType ARGUMENT_EXPECTED = translated( "argument.computercraft.argument_expected" );
|
|
|
|
private static SimpleCommandExceptionType translated( String key )
|
|
{
|
|
return new SimpleCommandExceptionType( new TextComponentTranslation( key ) );
|
|
}
|
|
|
|
private static DynamicCommandExceptionType translated1( String key )
|
|
{
|
|
return new DynamicCommandExceptionType( x -> new TextComponentTranslation( key, x ) );
|
|
}
|
|
|
|
private static Dynamic2CommandExceptionType translated2( String key )
|
|
{
|
|
return new Dynamic2CommandExceptionType( ( x, y ) -> new TextComponentTranslation( key, x, y ) );
|
|
}
|
|
}
|