mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2024-06-16 10:09:55 +00:00
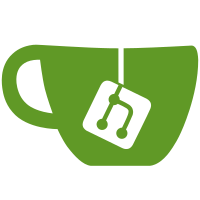
- Add a generic PermissionRegistry interface. This behaves similarly to our ShaderMod interface, searching all providers until it finds a compatible one. We could just make this part of the platform code instead, but this allows us to support multiple systems on Fabric, where things are less standardised. This interface behaves like a registry, rather than a straight `getPermission(node, player)` method, as Forge requires us to list our nodes up-front. - Add Forge (using the built-in system) and Fabric (using fabric-permissions-api) implementations of the above interface. - Register permission nodes for our commands, and use those instead. This does mean that the permissions check for the root /computercraft command now requires enumerating all child commands (and so potential does 7 permission lookups), but hopefully this isn't too bad in practice. - Remove UserLevel.OWNER - we never used this anywhere, and I can't imagine we'll want to in the future.
64 lines
1.7 KiB
Java
64 lines
1.7 KiB
Java
// SPDX-FileCopyrightText: 2017 The CC: Tweaked Developers
|
|
//
|
|
// SPDX-License-Identifier: MPL-2.0
|
|
|
|
package dan200.computercraft.shared.command;
|
|
|
|
import net.minecraft.commands.CommandSourceStack;
|
|
import net.minecraft.server.level.ServerPlayer;
|
|
|
|
import java.util.function.Predicate;
|
|
|
|
/**
|
|
* The level a user must be at in order to execute a command.
|
|
*/
|
|
public enum UserLevel implements Predicate<CommandSourceStack> {
|
|
/**
|
|
* Can only be used by ops.
|
|
*/
|
|
OP,
|
|
|
|
/**
|
|
* Can be used by any op, or the player in SSP.
|
|
*/
|
|
OWNER_OP,
|
|
|
|
/**
|
|
* Can be used by anyone.
|
|
*/
|
|
ANYONE;
|
|
|
|
public int toLevel() {
|
|
return switch (this) {
|
|
case OP, OWNER_OP -> 2;
|
|
case ANYONE -> 0;
|
|
};
|
|
}
|
|
|
|
@Override
|
|
public boolean test(CommandSourceStack source) {
|
|
if (this == ANYONE) return true;
|
|
if (this == OWNER_OP && isOwner(source)) return true;
|
|
return source.hasPermission(toLevel());
|
|
}
|
|
|
|
public boolean test(ServerPlayer source) {
|
|
if (this == ANYONE) return true;
|
|
if (this == OWNER_OP && isOwner(source)) return true;
|
|
return source.hasPermissions(toLevel());
|
|
}
|
|
|
|
public static boolean isOwner(CommandSourceStack source) {
|
|
var server = source.getServer();
|
|
var player = source.getPlayer();
|
|
return server.isDedicatedServer()
|
|
? source.getEntity() == null && source.hasPermission(4) && source.getTextName().equals("Server")
|
|
: player != null && server.isSingleplayerOwner(player.getGameProfile());
|
|
}
|
|
|
|
public static boolean isOwner(ServerPlayer player) {
|
|
var server = player.getServer();
|
|
return server != null && server.isSingleplayerOwner(player.getGameProfile());
|
|
}
|
|
}
|