mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2024-06-24 22:23:21 +00:00
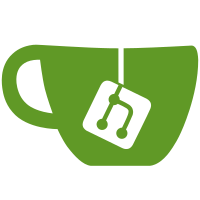
We now use illuaminate[1]'s linting facilities to check the rom and bios.lua for a couple of common bugs and other problems. Right now this doesn't detect any especially important bugs, though it has caught lots of small things (unused variables, some noisy code). In the future, the linter will grow in scope and features, which should allow us to be stricter and catch most issues. As a fun aside, we started off with ~150 bugs, and illuaminate was able to fix all but 30 of them, which is pretty neat. [1]: https://github.com/SquidDev/illuaminate
111 lines
3.4 KiB
Lua
111 lines
3.4 KiB
Lua
local expect = dofile("rom/modules/main/cc/expect.lua").expect
|
|
|
|
local native = peripheral
|
|
|
|
function getNames()
|
|
local tResults = {}
|
|
for _,sSide in ipairs( rs.getSides() ) do
|
|
if native.isPresent( sSide ) then
|
|
table.insert( tResults, sSide )
|
|
if native.getType( sSide ) == "modem" and not native.call( sSide, "isWireless" ) then
|
|
local tRemote = native.call( sSide, "getNamesRemote" )
|
|
for _,sName in ipairs( tRemote ) do
|
|
table.insert( tResults, sName )
|
|
end
|
|
end
|
|
end
|
|
end
|
|
return tResults
|
|
end
|
|
|
|
function isPresent( _sSide )
|
|
expect(1, _sSide, "string")
|
|
if native.isPresent( _sSide ) then
|
|
return true
|
|
end
|
|
for _,sSide in ipairs( rs.getSides() ) do
|
|
if native.getType( sSide ) == "modem" and not native.call( sSide, "isWireless" ) then
|
|
if native.call( sSide, "isPresentRemote", _sSide ) then
|
|
return true
|
|
end
|
|
end
|
|
end
|
|
return false
|
|
end
|
|
|
|
function getType( _sSide )
|
|
expect(1, _sSide, "string")
|
|
if native.isPresent( _sSide ) then
|
|
return native.getType( _sSide )
|
|
end
|
|
for _,sSide in ipairs( rs.getSides() ) do
|
|
if native.getType( sSide ) == "modem" and not native.call( sSide, "isWireless" ) then
|
|
if native.call( sSide, "isPresentRemote", _sSide ) then
|
|
return native.call( sSide, "getTypeRemote", _sSide )
|
|
end
|
|
end
|
|
end
|
|
return nil
|
|
end
|
|
|
|
function getMethods( _sSide )
|
|
expect(1, _sSide, "string")
|
|
if native.isPresent( _sSide ) then
|
|
return native.getMethods( _sSide )
|
|
end
|
|
for _,sSide in ipairs( rs.getSides() ) do
|
|
if native.getType( sSide ) == "modem" and not native.call( sSide, "isWireless" ) then
|
|
if native.call( sSide, "isPresentRemote", _sSide ) then
|
|
return native.call( sSide, "getMethodsRemote", _sSide )
|
|
end
|
|
end
|
|
end
|
|
return nil
|
|
end
|
|
|
|
function call( _sSide, _sMethod, ... )
|
|
expect(1, _sSide, "string")
|
|
expect(2, _sMethod, "string")
|
|
if native.isPresent( _sSide ) then
|
|
return native.call( _sSide, _sMethod, ... )
|
|
end
|
|
for _,sSide in ipairs( rs.getSides() ) do
|
|
if native.getType( sSide ) == "modem" and not native.call( sSide, "isWireless" ) then
|
|
if native.call( sSide, "isPresentRemote", _sSide ) then
|
|
return native.call( sSide, "callRemote", _sSide, _sMethod, ... )
|
|
end
|
|
end
|
|
end
|
|
return nil
|
|
end
|
|
|
|
function wrap( _sSide )
|
|
expect(1, _sSide, "string")
|
|
if peripheral.isPresent( _sSide ) then
|
|
local tMethods = peripheral.getMethods( _sSide )
|
|
local tResult = {}
|
|
for _,sMethod in ipairs( tMethods ) do
|
|
tResult[sMethod] = function( ... )
|
|
return peripheral.call( _sSide, sMethod, ... )
|
|
end
|
|
end
|
|
return tResult
|
|
end
|
|
return nil
|
|
end
|
|
|
|
function find( sType, fnFilter )
|
|
expect(1, sType, "string")
|
|
expect(2, fnFilter, "function", "nil")
|
|
local tResults = {}
|
|
for _,sName in ipairs( peripheral.getNames() ) do
|
|
if peripheral.getType( sName ) == sType then
|
|
local wrapped = peripheral.wrap( sName )
|
|
if fnFilter == nil or fnFilter( sName, wrapped ) then
|
|
table.insert( tResults, wrapped )
|
|
end
|
|
end
|
|
end
|
|
return table.unpack( tResults )
|
|
end
|