mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2024-06-25 22:53:22 +00:00
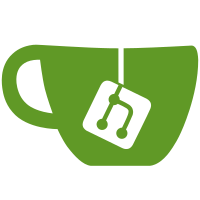
- Turtle and pocket computers provide a "creator mod id" based on their upgrade(s). We track which mod was active when the upgrade was registered, and use that to determine the owner. Technically we could use the RegistryLocation ID, but this is not always correct (such as Plethora's vanilla modules). - We show all upgraded turtles/pocket computers in JEI now, rather than just CC ones. - We provide a custom IRecipeRegistryPlugin for upgrades, which provides custom usage/recipes for any upgrade or upgraded item. We also hide our generated turtle/pocket computer recipes in order to prevent duplicates.
307 lines
9.4 KiB
Groovy
307 lines
9.4 KiB
Groovy
|
|
// For those who want the bleeding edge
|
|
buildscript {
|
|
repositories {
|
|
jcenter()
|
|
maven {
|
|
name = "forge"
|
|
url = "http://files.minecraftforge.net/maven"
|
|
}
|
|
}
|
|
dependencies {
|
|
classpath 'com.google.code.gson:gson:2.8.1'
|
|
classpath 'net.minecraftforge.gradle:ForgeGradle:2.3-SNAPSHOT'
|
|
classpath 'net.sf.proguard:proguard-gradle:6.1.0beta1'
|
|
classpath 'org.ajoberstar.grgit:grgit-gradle:3.0.0'
|
|
}
|
|
}
|
|
|
|
plugins {
|
|
id 'com.matthewprenger.cursegradle' version '1.0.10'
|
|
}
|
|
|
|
apply plugin: 'net.minecraftforge.gradle.forge'
|
|
apply plugin: 'org.ajoberstar.grgit'
|
|
apply plugin: 'maven-publish'
|
|
apply plugin: 'maven'
|
|
|
|
def mc_version = "1.12.2"
|
|
def main_version = "1.81.1"
|
|
version = "${mc_version}-${main_version}"
|
|
|
|
group = "org.squiddev"
|
|
archivesBaseName = "cc-tweaked"
|
|
|
|
minecraft {
|
|
version = "${mc_version}-14.23.4.2749"
|
|
runDir = "run"
|
|
replace '${version}', main_version
|
|
|
|
// the mappings can be changed at any time, and must be in the following format.
|
|
// snapshot_YYYYMMDD snapshot are built nightly.
|
|
// stable_# stables are built at the discretion of the MCP team.
|
|
// Use non-default mappings at your own risk. they may not allways work.
|
|
// simply re-run your setup task after changing the mappings to update your workspace.
|
|
mappings = "snapshot_20180724"
|
|
// makeObfSourceJar = false // an Srg named sources jar is made by default. uncomment this to disable.
|
|
}
|
|
|
|
repositories {
|
|
maven {
|
|
name = "JEI"
|
|
url = "http://dvs1.progwml6.com/files/maven"
|
|
}
|
|
maven {
|
|
name = "squiddev"
|
|
url = "https://squiddev.cc/maven"
|
|
}
|
|
|
|
ivy { artifactPattern "https://asie.pl/files/mods/Charset/LibOnly/[module]-[revision](-[classifier]).[ext]" }
|
|
maven { url "http://maven.amadornes.com/" }
|
|
}
|
|
|
|
configurations {
|
|
shade
|
|
compile.extendsFrom shade
|
|
deployerJars
|
|
}
|
|
|
|
dependencies {
|
|
deobfProvided "mezz.jei:jei_1.12.2:4.15.0.269:api"
|
|
deobfProvided "pl.asie:Charset-Lib:0.5.4.6"
|
|
deobfProvided "MCMultiPart2:MCMultiPart:2.5.3"
|
|
|
|
runtime "mezz.jei:jei_1.12.2:4.15.0.269"
|
|
|
|
shade 'org.squiddev:Cobalt:0.5.0-SNAPSHOT'
|
|
|
|
testImplementation 'org.junit.jupiter:junit-jupiter-api:5.1.0'
|
|
testRuntimeOnly 'org.junit.jupiter:junit-jupiter-engine:5.1.0'
|
|
|
|
deployerJars "org.apache.maven.wagon:wagon-ssh:3.0.0"
|
|
}
|
|
|
|
javadoc {
|
|
include "dan200/computercraft/api/**/*.java"
|
|
}
|
|
|
|
jar {
|
|
dependsOn javadoc
|
|
|
|
manifest {
|
|
attributes('FMLAT': 'computercraft_at.cfg')
|
|
}
|
|
|
|
from (sourceSets.main.allSource) {
|
|
include "dan200/computercraft/api/**/*.java"
|
|
}
|
|
|
|
from configurations.shade.collect { it.isDirectory() ? it : zipTree(it) }
|
|
}
|
|
|
|
import java.nio.charset.StandardCharsets
|
|
import java.nio.file.*
|
|
import java.util.zip.*
|
|
|
|
import com.google.gson.GsonBuilder
|
|
import com.google.gson.JsonElement
|
|
import org.ajoberstar.grgit.Grgit
|
|
import proguard.gradle.ProGuardTask
|
|
|
|
task proguard(type: ProGuardTask, dependsOn: jar) {
|
|
description "Removes unused shadowed classes from the jar"
|
|
group "compact"
|
|
|
|
injars jar.archivePath
|
|
outjars "${jar.archivePath.absolutePath.replace(".jar", "")}-min.jar"
|
|
|
|
// Add the main runtime jar and all non-shadowed dependencies
|
|
libraryjars "${System.getProperty('java.home')}/lib/rt.jar"
|
|
doFirst {
|
|
sourceSets.main.compileClasspath
|
|
.filter { !it.name.contains("Cobalt") }
|
|
.each { libraryjars it }
|
|
}
|
|
|
|
// We want to avoid as much obfuscation as possible. We're only doing this to shrink code size.
|
|
dontobfuscate; dontoptimize; keepattributes; keepparameternames
|
|
|
|
// Proguard will remove directories by default, but that breaks JarMount.
|
|
keepdirectories 'assets/computercraft/lua**'
|
|
|
|
// Preserve ComputerCraft classes - we only want to strip shadowed files.
|
|
keep 'class dan200.computercraft.** { *; }'
|
|
|
|
// Preserve the constructors in Cobalt library class, as we init them via reflection
|
|
keepclassmembers 'class org.squiddev.cobalt.lib.** { <init>(...); }'
|
|
}
|
|
|
|
task proguardMove(dependsOn: proguard) {
|
|
description "Replace the original jar with the minified version"
|
|
group "compact"
|
|
|
|
doLast {
|
|
Files.move(
|
|
file("${jar.archivePath.absolutePath.replace(".jar", "")}-min.jar").toPath(),
|
|
file(jar.archivePath).toPath(),
|
|
StandardCopyOption.REPLACE_EXISTING
|
|
)
|
|
}
|
|
}
|
|
|
|
reobfJar.dependsOn proguardMove
|
|
|
|
processResources {
|
|
inputs.property "version", main_version
|
|
inputs.property "mcversion", mc_version
|
|
|
|
def hash = 'none'
|
|
Set<String> contributors = []
|
|
try {
|
|
def grgit = Grgit.open(dir: '.')
|
|
hash = grgit.head().id
|
|
|
|
def blacklist = ['GitHub', 'dan200', 'Daniel Ratcliffe']
|
|
grgit.log().each {
|
|
if (!blacklist.contains(it.author.name)) contributors.add(it.author.name)
|
|
if (!blacklist.contains(it.committer.name)) contributors.add(it.committer.name)
|
|
}
|
|
} catch(Exception ignored) { }
|
|
|
|
inputs.property "commithash", hash
|
|
|
|
from(sourceSets.main.resources.srcDirs) {
|
|
include 'mcmod.info'
|
|
include 'assets/computercraft/lua/rom/help/credits.txt'
|
|
|
|
expand 'version': main_version,
|
|
'mcversion': mc_version,
|
|
'gitcontributors': contributors.sort(false, String.CASE_INSENSITIVE_ORDER).join('\n')
|
|
}
|
|
|
|
from(sourceSets.main.resources.srcDirs) {
|
|
exclude 'mcmod.info'
|
|
exclude 'assets/computercraft/lua/rom/help/credits.txt'
|
|
}
|
|
}
|
|
|
|
task compressJson(dependsOn: extractAnnotationsJar) {
|
|
group "compact"
|
|
description "Minifies all JSON files, stripping whitespace"
|
|
|
|
def jarPath = file(jar.archivePath)
|
|
|
|
def tempPath = File.createTempFile("input", ".jar", temporaryDir)
|
|
tempPath.deleteOnExit()
|
|
|
|
def gson = new GsonBuilder().create()
|
|
|
|
doLast {
|
|
// Copy over all files in the current jar to the new one, running json files from GSON. As pretty printing
|
|
// is turned off, they should be minified.
|
|
new ZipFile(jarPath).withCloseable { inJar ->
|
|
new ZipOutputStream(new BufferedOutputStream(new FileOutputStream(tempPath))).withCloseable { outJar ->
|
|
inJar.entries().each { entry ->
|
|
if(entry.directory) {
|
|
outJar.putNextEntry(entry)
|
|
} else if(!entry.name.endsWith(".json")) {
|
|
outJar.putNextEntry(entry)
|
|
inJar.getInputStream(entry).withCloseable { outJar << it }
|
|
} else {
|
|
ZipEntry newEntry = new ZipEntry(entry.name)
|
|
newEntry.setTime(entry.time)
|
|
outJar.putNextEntry(newEntry)
|
|
|
|
def element = inJar.getInputStream(entry).withCloseable { gson.fromJson(it.newReader("UTF8"), JsonElement.class) }
|
|
outJar.write(gson.toJson(element).getBytes(StandardCharsets.UTF_8))
|
|
}
|
|
}
|
|
|
|
}
|
|
}
|
|
|
|
// And replace the original jar again
|
|
Files.move(tempPath.toPath(), jarPath.toPath(), StandardCopyOption.REPLACE_EXISTING)
|
|
}
|
|
}
|
|
|
|
assemble.dependsOn compressJson
|
|
|
|
curseforge {
|
|
apiKey = project.hasProperty('curseForgeApiKey') ? project.curseForgeApiKey : ''
|
|
project {
|
|
id = '282001'
|
|
releaseType = 'release'
|
|
changelog = "Release notes can be found on the GitHub repository (https://github.com/SquidDev-CC/CC-Tweaked/releases/tag/v${project.version})."
|
|
}
|
|
}
|
|
|
|
publishing {
|
|
publications {
|
|
mavenJava(MavenPublication) {
|
|
from components.java
|
|
artifact sourceJar
|
|
}
|
|
}
|
|
}
|
|
|
|
uploadArchives {
|
|
repositories {
|
|
if(project.hasProperty('mavenUploadUrl')) {
|
|
mavenDeployer {
|
|
configuration = configurations.deployerJars
|
|
|
|
repository(url: project.property('mavenUploadUrl')) {
|
|
authentication(
|
|
userName: project.property('mavenUploadUser'),
|
|
privateKey: project.property('mavenUploadKey'))
|
|
}
|
|
|
|
pom.project {
|
|
name 'CC: Tweaked'
|
|
packaging 'jar'
|
|
description 'A fork of ComputerCraft which aims to provide earlier access to the more experimental and in-development features of the mod.'
|
|
url 'https://github.com/SquidDev-CC/CC-Tweaked'
|
|
|
|
scm {
|
|
url 'https://github.com/dan200/ComputerCraft.git'
|
|
}
|
|
|
|
issueManagement {
|
|
system 'github'
|
|
url 'https://github.com/dan200/ComputerCraft/issues'
|
|
}
|
|
|
|
licenses {
|
|
license {
|
|
name 'ComputerCraft Public License, Version 1.0'
|
|
url 'https://github.com/dan200/ComputerCraft/blob/master/LICENSE'
|
|
distribution 'repo'
|
|
}
|
|
}
|
|
}
|
|
|
|
pom.whenConfigured { pom ->
|
|
pom.dependencies.clear()
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
test {
|
|
useJUnitPlatform()
|
|
testLogging {
|
|
events "passed", "skipped", "failed"
|
|
}
|
|
}
|
|
|
|
gradle.projectsEvaluated {
|
|
tasks.withType(JavaCompile) {
|
|
options.compilerArgs << "-Xlint"
|
|
}
|
|
}
|
|
|
|
runClient.outputs.upToDateWhen { false }
|
|
runServer.outputs.upToDateWhen { false }
|