mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2024-06-17 10:50:01 +00:00
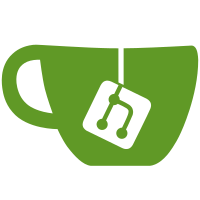
- Separate FileMount into separate FileMount and WritableFileMount classes. This separates the (relatively simple) read-only code from the (soon to be even more complex) read/write code. It also allows you to create read-only mounts which don't bother with filesystem accounting, which is nice. - Make openForWrite/openForAppend always return a SeekableFileHandle. Appendable files still cannot be seeked within, but that check is now done on the FS side. - Refactor the various mount tests to live in test contract interfaces, allowing us to reuse them between mounts. - Clean up our error handling a little better. (Most) file-specific code has been moved to FileMount, and ArchiveMount-derived classes now throw correct path-localised exceptions.
62 lines
1.9 KiB
Java
62 lines
1.9 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2022. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
package dan200.computercraft.test.core;
|
|
|
|
import javax.annotation.Nullable;
|
|
import java.util.ArrayDeque;
|
|
import java.util.Deque;
|
|
import java.util.Objects;
|
|
|
|
/**
|
|
* An {@link AutoCloseable} implementation which can be used to combine other [AutoCloseable] instances.
|
|
* <p>
|
|
* Values which implement {@link AutoCloseable} can be dynamically registered with [CloseScope.add]. When the scope is
|
|
* closed, each value is closed in the opposite order.
|
|
* <p>
|
|
* This is largely intended for cases where it's not appropriate to nest try-with-resources blocks, for instance when
|
|
* nested would be too deep or when objects are dynamically created.
|
|
*/
|
|
public class CloseScope implements AutoCloseable {
|
|
private final Deque<AutoCloseable> toClose = new ArrayDeque<>();
|
|
|
|
/**
|
|
* Add a value to be closed when this scope exists.
|
|
*
|
|
* @param value The value to be closed.
|
|
* @param <T> The type of the provided value.
|
|
* @return The provided value.
|
|
*/
|
|
public <T extends AutoCloseable> T add(T value) {
|
|
Objects.requireNonNull(value, "Value cannot be null");
|
|
toClose.add(value);
|
|
return value;
|
|
}
|
|
|
|
@Override
|
|
public void close() throws Exception {
|
|
close(null);
|
|
}
|
|
|
|
public void close(@Nullable Exception baseException) throws Exception {
|
|
while (true) {
|
|
var close = toClose.pollLast();
|
|
if (close == null) break;
|
|
|
|
try {
|
|
close.close();
|
|
} catch (Exception e) {
|
|
if (baseException == null) {
|
|
baseException = e;
|
|
} else {
|
|
baseException.addSuppressed(e);
|
|
}
|
|
}
|
|
}
|
|
|
|
if (baseException != null) throw baseException;
|
|
}
|
|
}
|