mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2024-06-16 18:19:55 +00:00
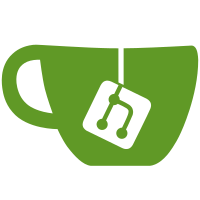
- Separate FileMount into separate FileMount and WritableFileMount classes. This separates the (relatively simple) read-only code from the (soon to be even more complex) read/write code. It also allows you to create read-only mounts which don't bother with filesystem accounting, which is nice. - Make openForWrite/openForAppend always return a SeekableFileHandle. Appendable files still cannot be seeked within, but that check is now done on the FS side. - Refactor the various mount tests to live in test contract interfaces, allowing us to reuse them between mounts. - Clean up our error handling a little better. (Most) file-specific code has been moved to FileMount, and ArchiveMount-derived classes now throw correct path-localised exceptions.
46 lines
1.2 KiB
Java
46 lines
1.2 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2022. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
package dan200.computercraft.core.computer;
|
|
|
|
import dan200.computercraft.api.filesystem.WritableMount;
|
|
import dan200.computercraft.core.filesystem.WritableFileMount;
|
|
import dan200.computercraft.core.metrics.MetricsObserver;
|
|
|
|
import javax.annotation.Nullable;
|
|
|
|
public interface ComputerEnvironment {
|
|
/**
|
|
* Get the current in-game day.
|
|
*
|
|
* @return The current day.
|
|
*/
|
|
int getDay();
|
|
|
|
/**
|
|
* Get the current in-game time of day.
|
|
*
|
|
* @return The current time.
|
|
*/
|
|
double getTimeOfDay();
|
|
|
|
/**
|
|
* Get the {@link MetricsObserver} for this computer. This should be constant for the duration of this
|
|
* {@link ComputerEnvironment}.
|
|
*
|
|
* @return This computer's {@link MetricsObserver}.
|
|
*/
|
|
MetricsObserver getMetrics();
|
|
|
|
/**
|
|
* Construct the mount for this computer's user-writable data.
|
|
*
|
|
* @return The constructed mount or {@code null} if the mount could not be created.
|
|
* @see WritableFileMount
|
|
*/
|
|
@Nullable
|
|
WritableMount createRootMount();
|
|
}
|