mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2025-07-03 02:22:51 +00:00
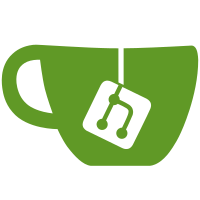
- Separate FileMount into separate FileMount and WritableFileMount classes. This separates the (relatively simple) read-only code from the (soon to be even more complex) read/write code. It also allows you to create read-only mounts which don't bother with filesystem accounting, which is nice. - Make openForWrite/openForAppend always return a SeekableFileHandle. Appendable files still cannot be seeked within, but that check is now done on the FS side. - Refactor the various mount tests to live in test contract interfaces, allowing us to reuse them between mounts. - Clean up our error handling a little better. (Most) file-specific code has been moved to FileMount, and ArchiveMount-derived classes now throw correct path-localised exceptions.
64 lines
2.3 KiB
Java
64 lines
2.3 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2022. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
package dan200.computercraft.shared.computer.core;
|
|
|
|
import com.google.common.io.MoreFiles;
|
|
import com.google.common.io.RecursiveDeleteOption;
|
|
import dan200.computercraft.api.filesystem.Mount;
|
|
import dan200.computercraft.test.core.CloseScope;
|
|
import dan200.computercraft.test.core.filesystem.MountContract;
|
|
import net.minecraft.Util;
|
|
import net.minecraft.server.packs.PackType;
|
|
import net.minecraft.server.packs.PathPackResources;
|
|
import net.minecraft.server.packs.resources.ReloadableResourceManager;
|
|
import net.minecraft.util.Unit;
|
|
import org.junit.jupiter.api.AfterEach;
|
|
|
|
import java.io.IOException;
|
|
import java.nio.file.Files;
|
|
import java.util.List;
|
|
import java.util.concurrent.CompletableFuture;
|
|
import java.util.concurrent.ExecutionException;
|
|
|
|
public class ResourceMountTest implements MountContract {
|
|
private final CloseScope toClose = new CloseScope();
|
|
|
|
@Override
|
|
public Mount createSkeleton() throws IOException {
|
|
var path = Files.createTempDirectory("cctweaked-test");
|
|
toClose.add(() -> MoreFiles.deleteRecursively(path, RecursiveDeleteOption.ALLOW_INSECURE));
|
|
|
|
Files.createDirectories(path.resolve("data/computercraft/rom/dir"));
|
|
try (var writer = Files.newBufferedWriter(path.resolve("data/computercraft/rom/dir/file.lua"))) {
|
|
writer.write("print('testing')");
|
|
}
|
|
Files.newBufferedWriter(path.resolve("data/computercraft/rom/f.lua")).close();
|
|
|
|
var manager = new ReloadableResourceManager(PackType.SERVER_DATA);
|
|
var reload = manager.createReload(Util.backgroundExecutor(), Util.backgroundExecutor(), CompletableFuture.completedFuture(Unit.INSTANCE), List.of(
|
|
new PathPackResources("resources", path, false)
|
|
));
|
|
|
|
try {
|
|
reload.done().get();
|
|
} catch (InterruptedException | ExecutionException e) {
|
|
throw new RuntimeException("Failed to load resources", e);
|
|
}
|
|
|
|
return new ResourceMount("computercraft", "rom", manager);
|
|
}
|
|
|
|
@Override
|
|
public boolean hasFileTimes() {
|
|
return false;
|
|
}
|
|
|
|
@AfterEach
|
|
public void after() throws Exception {
|
|
toClose.close();
|
|
}
|
|
}
|