mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2024-06-23 13:43:22 +00:00
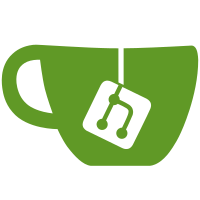
Look, I originally had this split into several commits, but lots of other cleanups got mixed in. I then backported some of the cleanups to 1.12, did other tidy ups there, and eventually the web of merges was unreadable. Yes, this is a horrible mess, but it's still nicer than it was. Anyway, changes: - Flatten everything. For instance, there are now three instances of BlockComputer, two BlockTurtle, ItemPocketComputer. There's also no more BlockPeripheral (thank heavens) - there's separate block classes for each peripheral type. - Remove pretty much all legacy code. As we're breaking world compatibility anyway, we can remove all the code to load worlds from 1.4 days. - The command system is largely rewriten to take advantage of 1.13's new system. It's very fancy! - WidgetTerminal now uses Minecraft's "GUI listener" system. - BREAKING CHANGE: All the codes in keys.lua are different, due to the move to LWJGL 3. Hopefully this won't have too much of an impact. I don't want to map to the old key codes on the Java side, as there always ends up being small but slight inconsistencies. IMO it's better to make a clean break - people should be using keys rather than hard coding the constants anyway. - commands.list now allows fetching sub-commands. The ROM has already been updated to allow fancy usage such as commands.time.set("noon"). - Turtles, modems and cables can be waterlogged.
84 lines
3.1 KiB
Java
84 lines
3.1 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2019. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
|
|
package dan200.computercraft.shared.turtle.core;
|
|
|
|
import com.google.common.collect.ImmutableMap;
|
|
import dan200.computercraft.api.turtle.ITurtleAccess;
|
|
import dan200.computercraft.api.turtle.ITurtleCommand;
|
|
import dan200.computercraft.api.turtle.TurtleCommandResult;
|
|
import dan200.computercraft.api.turtle.event.TurtleBlockEvent;
|
|
import net.minecraft.block.Block;
|
|
import net.minecraft.block.state.IBlockState;
|
|
import net.minecraft.state.IProperty;
|
|
import net.minecraft.util.EnumFacing;
|
|
import net.minecraft.util.math.BlockPos;
|
|
import net.minecraft.world.World;
|
|
import net.minecraftforge.common.MinecraftForge;
|
|
import net.minecraftforge.registries.ForgeRegistries;
|
|
|
|
import javax.annotation.Nonnull;
|
|
import java.util.HashMap;
|
|
import java.util.Map;
|
|
|
|
public class TurtleInspectCommand implements ITurtleCommand
|
|
{
|
|
private final InteractDirection direction;
|
|
|
|
public TurtleInspectCommand( InteractDirection direction )
|
|
{
|
|
this.direction = direction;
|
|
}
|
|
|
|
@Nonnull
|
|
@Override
|
|
public TurtleCommandResult execute( @Nonnull ITurtleAccess turtle )
|
|
{
|
|
// Get world direction from direction
|
|
EnumFacing direction = this.direction.toWorldDir( turtle );
|
|
|
|
// Check if thing in front is air or not
|
|
World world = turtle.getWorld();
|
|
BlockPos oldPosition = turtle.getPosition();
|
|
BlockPos newPosition = oldPosition.offset( direction );
|
|
|
|
IBlockState state = world.getBlockState( newPosition );
|
|
if( state.getBlock().isAir( state, world, newPosition ) )
|
|
{
|
|
return TurtleCommandResult.failure( "No block to inspect" );
|
|
}
|
|
|
|
Block block = state.getBlock();
|
|
String name = ForgeRegistries.BLOCKS.getKey( block ).toString();
|
|
|
|
Map<String, Object> table = new HashMap<>();
|
|
table.put( "name", name );
|
|
|
|
Map<Object, Object> stateTable = new HashMap<>();
|
|
for( ImmutableMap.Entry<IProperty<?>, ? extends Comparable<?>> entry : state.getValues().entrySet() )
|
|
{
|
|
IProperty<?> property = entry.getKey();
|
|
stateTable.put( property.getName(), getPropertyValue( property, entry.getValue() ) );
|
|
}
|
|
table.put( "state", stateTable );
|
|
|
|
// Fire the event, exiting if it is cancelled
|
|
TurtlePlayer turtlePlayer = TurtlePlaceCommand.createPlayer( turtle, oldPosition, direction );
|
|
TurtleBlockEvent.Inspect event = new TurtleBlockEvent.Inspect( turtle, turtlePlayer, world, newPosition, state, table );
|
|
if( MinecraftForge.EVENT_BUS.post( event ) ) return TurtleCommandResult.failure( event.getFailureMessage() );
|
|
|
|
return TurtleCommandResult.success( new Object[] { table } );
|
|
|
|
}
|
|
|
|
@SuppressWarnings( { "unchecked", "rawtypes" } )
|
|
private static Object getPropertyValue( IProperty property, Comparable value )
|
|
{
|
|
if( value instanceof String || value instanceof Number || value instanceof Boolean ) return value;
|
|
return property.getName( value );
|
|
}
|
|
}
|