mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2024-06-23 13:43:22 +00:00
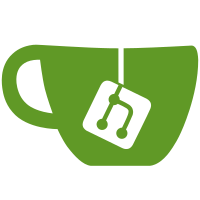
- Fire all the appropriate Forge hooks - Crafting will now attempt to craft one item at a time in a loop, instead of multiplying the resulting stack by the number of crafts. This means we function as expected on recipes which consume durability instead. - Cache the recipe between crafting and getting the remainder (and each craft loop). This should reduce any performance hit we would otherwise get.
53 lines
1.8 KiB
Java
53 lines
1.8 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2019. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
|
|
package dan200.computercraft.shared.turtle.core;
|
|
|
|
import dan200.computercraft.api.turtle.ITurtleAccess;
|
|
import dan200.computercraft.api.turtle.ITurtleCommand;
|
|
import dan200.computercraft.api.turtle.TurtleAnimation;
|
|
import dan200.computercraft.api.turtle.TurtleCommandResult;
|
|
import dan200.computercraft.shared.turtle.upgrades.TurtleInventoryCrafting;
|
|
import dan200.computercraft.shared.util.InventoryUtil;
|
|
import dan200.computercraft.shared.util.WorldUtil;
|
|
import net.minecraft.item.ItemStack;
|
|
|
|
import javax.annotation.Nonnull;
|
|
import java.util.List;
|
|
|
|
public class TurtleCraftCommand implements ITurtleCommand
|
|
{
|
|
private final int limit;
|
|
|
|
public TurtleCraftCommand( int limit )
|
|
{
|
|
this.limit = limit;
|
|
}
|
|
|
|
@Nonnull
|
|
@Override
|
|
public TurtleCommandResult execute( @Nonnull ITurtleAccess turtle )
|
|
{
|
|
// Craft the item
|
|
TurtleInventoryCrafting crafting = new TurtleInventoryCrafting( turtle );
|
|
List<ItemStack> results = crafting.doCrafting( turtle.getWorld(), limit );
|
|
if( results == null ) return TurtleCommandResult.failure( "No matching recipes" );
|
|
|
|
// Store or drop any remainders
|
|
for( ItemStack stack : results )
|
|
{
|
|
ItemStack remainder = InventoryUtil.storeItems( stack, turtle.getItemHandler(), turtle.getSelectedSlot() );
|
|
if( !remainder.isEmpty() )
|
|
{
|
|
WorldUtil.dropItemStack( remainder, turtle.getWorld(), turtle.getPosition(), turtle.getDirection() );
|
|
}
|
|
}
|
|
|
|
if( !results.isEmpty() ) turtle.playAnimation( TurtleAnimation.Wait );
|
|
return TurtleCommandResult.success();
|
|
}
|
|
}
|