mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2025-07-03 10:32:52 +00:00
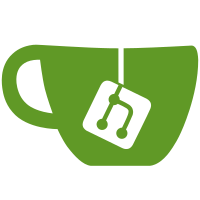
Look, I originally had this split into several commits, but lots of other cleanups got mixed in. I then backported some of the cleanups to 1.12, did other tidy ups there, and eventually the web of merges was unreadable. Yes, this is a horrible mess, but it's still nicer than it was. Anyway, changes: - Flatten everything. For instance, there are now three instances of BlockComputer, two BlockTurtle, ItemPocketComputer. There's also no more BlockPeripheral (thank heavens) - there's separate block classes for each peripheral type. - Remove pretty much all legacy code. As we're breaking world compatibility anyway, we can remove all the code to load worlds from 1.4 days. - The command system is largely rewriten to take advantage of 1.13's new system. It's very fancy! - WidgetTerminal now uses Minecraft's "GUI listener" system. - BREAKING CHANGE: All the codes in keys.lua are different, due to the move to LWJGL 3. Hopefully this won't have too much of an impact. I don't want to map to the old key codes on the Java side, as there always ends up being small but slight inconsistencies. IMO it's better to make a clean break - people should be using keys rather than hard coding the constants anyway. - commands.list now allows fetching sub-commands. The ROM has already been updated to allow fancy usage such as commands.time.set("noon"). - Turtles, modems and cables can be waterlogged.
145 lines
4.4 KiB
Java
145 lines
4.4 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2019. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
|
|
package dan200.computercraft.shared.peripheral.modem.wired;
|
|
|
|
import dan200.computercraft.ComputerCraft;
|
|
import dan200.computercraft.api.peripheral.IPeripheral;
|
|
import dan200.computercraft.shared.Peripherals;
|
|
import dan200.computercraft.shared.util.IDAssigner;
|
|
import net.minecraft.block.Block;
|
|
import net.minecraft.nbt.NBTTagCompound;
|
|
import net.minecraft.util.EnumFacing;
|
|
import net.minecraft.util.math.BlockPos;
|
|
import net.minecraft.world.World;
|
|
import net.minecraftforge.common.util.Constants;
|
|
|
|
import javax.annotation.Nonnull;
|
|
import javax.annotation.Nullable;
|
|
import java.util.Collections;
|
|
import java.util.Map;
|
|
|
|
/**
|
|
* Represents a local peripheral exposed on the wired network
|
|
*
|
|
* This is responsible for getting the peripheral in world, tracking id and type and determining whether
|
|
* it has changed.
|
|
*/
|
|
public final class WiredModemLocalPeripheral
|
|
{
|
|
private static final String NBT_PERIPHERAL_TYPE = "PeripheralType";
|
|
private static final String NBT_PERIPHERAL_ID = "PeripheralId";
|
|
|
|
private int id;
|
|
private String type;
|
|
|
|
private IPeripheral peripheral;
|
|
|
|
/**
|
|
* Attach a new peripheral from the world
|
|
*
|
|
* @param world The world to search in
|
|
* @param origin The position to search from
|
|
* @param direction The direction so search in
|
|
* @return Whether the peripheral changed.
|
|
*/
|
|
public boolean attach( @Nonnull World world, @Nonnull BlockPos origin, @Nonnull EnumFacing direction )
|
|
{
|
|
IPeripheral oldPeripheral = peripheral;
|
|
IPeripheral peripheral = this.peripheral = getPeripheralFrom( world, origin, direction );
|
|
|
|
if( peripheral == null )
|
|
{
|
|
return oldPeripheral != null;
|
|
}
|
|
else
|
|
{
|
|
String type = peripheral.getType();
|
|
int id = this.id;
|
|
|
|
if( id > 0 && this.type == null )
|
|
{
|
|
// If we had an ID but no type, then just set the type.
|
|
this.type = type;
|
|
}
|
|
else if( id < 0 || !type.equals( this.type ) )
|
|
{
|
|
this.type = type;
|
|
this.id = IDAssigner.getNextId( "peripheral." + type );
|
|
}
|
|
|
|
return oldPeripheral == null || !oldPeripheral.equals( peripheral );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Detach the current peripheral
|
|
*
|
|
* @return Whether the peripheral changed
|
|
*/
|
|
public boolean detach()
|
|
{
|
|
if( peripheral == null ) return false;
|
|
peripheral = null;
|
|
return true;
|
|
}
|
|
|
|
@Nullable
|
|
public String getConnectedName()
|
|
{
|
|
return peripheral != null ? type + "_" + id : null;
|
|
}
|
|
|
|
@Nullable
|
|
public IPeripheral getPeripheral()
|
|
{
|
|
return peripheral;
|
|
}
|
|
|
|
public boolean hasPeripheral()
|
|
{
|
|
return peripheral != null;
|
|
}
|
|
|
|
public void extendMap( @Nonnull Map<String, IPeripheral> peripherals )
|
|
{
|
|
if( peripheral != null ) peripherals.put( type + "_" + id, peripheral );
|
|
}
|
|
|
|
public Map<String, IPeripheral> toMap()
|
|
{
|
|
return peripheral == null
|
|
? Collections.emptyMap()
|
|
: Collections.singletonMap( type + "_" + id, peripheral );
|
|
}
|
|
|
|
public void write( @Nonnull NBTTagCompound tag, @Nonnull String suffix )
|
|
{
|
|
if( id >= 0 ) tag.putInt( NBT_PERIPHERAL_ID + suffix, id );
|
|
if( type != null ) tag.putString( NBT_PERIPHERAL_TYPE + suffix, type );
|
|
}
|
|
|
|
public void read( @Nonnull NBTTagCompound tag, @Nonnull String suffix )
|
|
{
|
|
id = tag.contains( NBT_PERIPHERAL_ID + suffix, Constants.NBT.TAG_ANY_NUMERIC )
|
|
? tag.getInt( NBT_PERIPHERAL_ID + suffix ) : -1;
|
|
|
|
type = tag.contains( NBT_PERIPHERAL_TYPE + suffix, Constants.NBT.TAG_STRING )
|
|
? tag.getString( NBT_PERIPHERAL_TYPE + suffix ) : null;
|
|
}
|
|
|
|
private static IPeripheral getPeripheralFrom( World world, BlockPos pos, EnumFacing direction )
|
|
{
|
|
BlockPos offset = pos.offset( direction );
|
|
|
|
Block block = world.getBlockState( offset ).getBlock();
|
|
if( block == ComputerCraft.Blocks.wiredModemFull || block == ComputerCraft.Blocks.cable ) return null;
|
|
|
|
IPeripheral peripheral = Peripherals.getPeripheral( world, offset, direction.getOpposite() );
|
|
return peripheral instanceof WiredModemPeripheral ? null : peripheral;
|
|
}
|
|
}
|