mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2025-07-05 03:22:53 +00:00
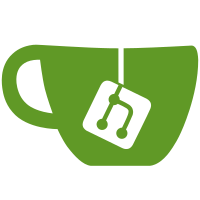
Look, I originally had this split into several commits, but lots of other cleanups got mixed in. I then backported some of the cleanups to 1.12, did other tidy ups there, and eventually the web of merges was unreadable. Yes, this is a horrible mess, but it's still nicer than it was. Anyway, changes: - Flatten everything. For instance, there are now three instances of BlockComputer, two BlockTurtle, ItemPocketComputer. There's also no more BlockPeripheral (thank heavens) - there's separate block classes for each peripheral type. - Remove pretty much all legacy code. As we're breaking world compatibility anyway, we can remove all the code to load worlds from 1.4 days. - The command system is largely rewriten to take advantage of 1.13's new system. It's very fancy! - WidgetTerminal now uses Minecraft's "GUI listener" system. - BREAKING CHANGE: All the codes in keys.lua are different, due to the move to LWJGL 3. Hopefully this won't have too much of an impact. I don't want to map to the old key codes on the Java side, as there always ends up being small but slight inconsistencies. IMO it's better to make a clean break - people should be using keys rather than hard coding the constants anyway. - commands.list now allows fetching sub-commands. The ROM has already been updated to allow fancy usage such as commands.time.set("noon"). - Turtles, modems and cables can be waterlogged.
101 lines
3.5 KiB
Java
101 lines
3.5 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2019. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
|
|
package dan200.computercraft.shared.peripheral.modem.wired;
|
|
|
|
import com.google.common.collect.ImmutableMap;
|
|
import dan200.computercraft.shared.peripheral.modem.ModemShapes;
|
|
import dan200.computercraft.shared.util.DirectionUtil;
|
|
import net.minecraft.block.state.IBlockState;
|
|
import net.minecraft.util.EnumFacing;
|
|
import net.minecraft.util.math.shapes.VoxelShape;
|
|
import net.minecraft.util.math.shapes.VoxelShapes;
|
|
|
|
import java.util.EnumMap;
|
|
|
|
import static dan200.computercraft.shared.peripheral.modem.wired.BlockCable.*;
|
|
|
|
public final class CableShapes
|
|
{
|
|
private static final double MIN = 0.375;
|
|
private static final double MAX = 1 - MIN;
|
|
|
|
private static final VoxelShape SHAPE_CABLE_CORE = VoxelShapes.create( MIN, MIN, MIN, MAX, MAX, MAX );
|
|
private static final EnumMap<EnumFacing, VoxelShape> SHAPE_CABLE_ARM =
|
|
new EnumMap<>( new ImmutableMap.Builder<EnumFacing, VoxelShape>()
|
|
.put( EnumFacing.DOWN, VoxelShapes.create( MIN, 0, MIN, MAX, MIN, MAX ) )
|
|
.put( EnumFacing.UP, VoxelShapes.create( MIN, MAX, MIN, MAX, 1, MAX ) )
|
|
.put( EnumFacing.NORTH, VoxelShapes.create( MIN, MIN, 0, MAX, MAX, MIN ) )
|
|
.put( EnumFacing.SOUTH, VoxelShapes.create( MIN, MIN, MAX, MAX, MAX, 1 ) )
|
|
.put( EnumFacing.WEST, VoxelShapes.create( 0, MIN, MIN, MIN, MAX, MAX ) )
|
|
.put( EnumFacing.EAST, VoxelShapes.create( MAX, MIN, MIN, 1, MAX, MAX ) )
|
|
.build()
|
|
);
|
|
|
|
private static final VoxelShape[] SHAPES = new VoxelShape[(1 << 6) * 7];
|
|
private static final VoxelShape[] CABLE_SHAPES = new VoxelShape[1 << 6];
|
|
|
|
private CableShapes()
|
|
{
|
|
}
|
|
|
|
private static int getCableIndex( IBlockState state )
|
|
{
|
|
int index = 0;
|
|
for( EnumFacing facing : DirectionUtil.FACINGS )
|
|
{
|
|
if( state.get( CONNECTIONS.get( facing ) ) ) index |= 1 << facing.ordinal();
|
|
}
|
|
|
|
return index;
|
|
}
|
|
|
|
private static VoxelShape getCableShape( int index )
|
|
{
|
|
VoxelShape shape = CABLE_SHAPES[index];
|
|
if( shape != null ) return shape;
|
|
|
|
shape = SHAPE_CABLE_CORE;
|
|
for( EnumFacing facing : DirectionUtil.FACINGS )
|
|
{
|
|
if( (index & (1 << facing.ordinal())) != 0 )
|
|
{
|
|
shape = VoxelShapes.or( shape, SHAPE_CABLE_ARM.get( facing ) );
|
|
}
|
|
}
|
|
|
|
return CABLE_SHAPES[index] = shape;
|
|
}
|
|
|
|
public static VoxelShape getCableShape( IBlockState state )
|
|
{
|
|
if( !state.get( CABLE ) ) return VoxelShapes.empty();
|
|
return getCableShape( getCableIndex( state ) );
|
|
}
|
|
|
|
public static VoxelShape getModemShape( IBlockState state )
|
|
{
|
|
EnumFacing facing = state.get( MODEM ).getFacing();
|
|
return facing == null ? VoxelShapes.empty() : ModemShapes.getBounds( facing );
|
|
}
|
|
|
|
public static VoxelShape getShape( IBlockState state )
|
|
{
|
|
EnumFacing facing = state.get( MODEM ).getFacing();
|
|
if( !state.get( CABLE ) ) return getModemShape( state );
|
|
|
|
int cableIndex = getCableIndex( state );
|
|
int index = cableIndex + ((facing == null ? 0 : facing.ordinal() + 1) << 6);
|
|
|
|
VoxelShape shape = SHAPES[index];
|
|
if( shape != null ) return shape;
|
|
|
|
shape = getCableShape( cableIndex );
|
|
if( facing != null ) shape = VoxelShapes.or( shape, ModemShapes.getBounds( facing ) );
|
|
return SHAPES[index] = shape;
|
|
}
|
|
}
|