mirror of
https://github.com/SquidDev-CC/CC-Tweaked
synced 2025-07-04 11:02:54 +00:00
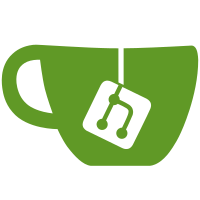
OK, so let's get this out of the way, there's some actual changes mixed in here too. I'm really sorry: - Turtles can now not be renamed with unnamed item tags (previously it would clear the name, this seemed a little unideal). - commands.getBlock(s)Data will also include NBT. Now, onto the horror story which is these inspection changes: - Make a lot of methods static - Typo fixes - Make utility classes final + private constructor - Lots of reformatting (ifs -> ternary, invert control flow, etc...) - ??? - Profit! I'm so going to regret this - can pretty much guarantee this is going to break something.
54 lines
1.7 KiB
Java
54 lines
1.7 KiB
Java
/*
|
|
* This file is part of ComputerCraft - http://www.computercraft.info
|
|
* Copyright Daniel Ratcliffe, 2011-2019. Do not distribute without permission.
|
|
* Send enquiries to dratcliffe@gmail.com
|
|
*/
|
|
|
|
package dan200.computercraft.core.filesystem;
|
|
|
|
import javax.annotation.Nonnull;
|
|
import java.io.Closeable;
|
|
import java.io.IOException;
|
|
import java.lang.ref.ReferenceQueue;
|
|
import java.lang.ref.WeakReference;
|
|
|
|
/**
|
|
* An alternative closeable implementation that will free up resources in the filesystem.
|
|
*
|
|
* The {@link FileSystem} maps weak references of this to its underlying object. If the wrapper has been disposed of
|
|
* (say, the Lua object referencing it has gone), then the wrapped object will be closed by the filesystem.
|
|
*
|
|
* Closing this will stop the filesystem tracking it, reducing the current descriptor count.
|
|
*
|
|
* In an ideal world, we'd just wrap the closeable. However, as we do some {@code instanceof} checks
|
|
* on the stream, it's not really possible as it'd require numerous instances.
|
|
*
|
|
* @param <T> The type of writer or channel to wrap.
|
|
*/
|
|
public class FileSystemWrapper<T extends Closeable> implements Closeable
|
|
{
|
|
private final FileSystem fileSystem;
|
|
private final ChannelWrapper<T> closeable;
|
|
final WeakReference<FileSystemWrapper<?>> self;
|
|
|
|
FileSystemWrapper( FileSystem fileSystem, ChannelWrapper<T> closeable, ReferenceQueue<FileSystemWrapper<?>> queue )
|
|
{
|
|
this.fileSystem = fileSystem;
|
|
this.closeable = closeable;
|
|
self = new WeakReference<>( this, queue );
|
|
}
|
|
|
|
@Override
|
|
public void close() throws IOException
|
|
{
|
|
fileSystem.removeFile( this );
|
|
closeable.close();
|
|
}
|
|
|
|
@Nonnull
|
|
public T get()
|
|
{
|
|
return closeable.get();
|
|
}
|
|
}
|